Main script to run iGFA¶
import os
import sys
import warnings
import pickle
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import pyomo.environ as pyo
from pyomo.contrib.iis import compute_infeasibility_explanation
warnings.filterwarnings("ignore", "use_inf_as_na") # Generated by seaborn
sys.path.insert(0, '/home/cabsel/gfa/')
from gfapy.model import summarize_instance
from gfapy.plotter import plot_fig, plot_meas, plot_perturb
from gfapy.igfa_fitflux import parse_model, res_plotter
/home/cabsel/gfa/gfapy/model.py:3: TqdmExperimentalWarning: Using `tqdm.autonotebook.tqdm` in notebook mode. Use `tqdm.tqdm` instead to force console mode (e.g. in jupyter console)
from tqdm.autonotebook import tqdm
mainDir = '/home/cabsel/gfa/'
inputDir = os.path.join(mainDir, 'inputfiles')
Read GFA model from Excel¶
rxn_models = pd.read_excel(os.path.join(inputDir, 'Lee et al', 'Leeetal_model.xlsx'), sheet_name=None)
stoich_data = rxn_models['Stoichiometry'].iloc[1:, :].set_index("Glycoforms")
rxn_meta = rxn_models['Enzyme-Reaction Relations'].set_index("Reaction ID")
met_meta_cols = sorted(set(stoich_data.columns) - set(rxn_meta.index))
met_meta = stoich_data[met_meta_cols].copy()
stoich_data = stoich_data.drop(columns=met_meta_cols)
display(stoich_data)
display(met_meta)
display(rxn_meta)
Ent_Man7 | Ex_Man7 | Ex_Man6 | Ex_Man5 | Ex_G0-GlcNAc | Ex_G0 | Ex_G0F | Ex_G0F-GlcNAc | Ex_G0F+GlcNAc | Ex_G1a/b | ... | R8 | R6 | R7 | R9 | R10 | R11 | R12 | R13 | R14 | R15 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Glycoforms | |||||||||||||||||||||
Man7 | 1.0 | -1.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Man6 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Man5 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
G0-GlcNAc | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | -1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
G0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | -1 | 0 | 0 | -1 | 0 | 0 | 0 | 0 | 0 |
G0F | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | 0.0 | ... | -1 | 1 | 0 | 0 | 0 | -1 | 0 | 0 | 0 | 0 |
G0F-GlcNAc | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | 0.0 | ... | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
G0F+GlcNAc | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | 0.0 | ... | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
G1a/b | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | -1.0 | ... | 0 | 0 | 0 | -1 | 1 | 0 | 0 | 0 | 0 | 0 |
G1Fa/b | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 1 | 0 | 1 | -1 | 0 | -1 | 0 |
G2F | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | -1 |
A1G1F | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | -1 | 1 | 0 |
A1G2F | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 1 |
13 rows × 29 columns
Compartments | Structure | |
---|---|---|
Glycoforms | ||
Man7 | cis_golgi | {Man(1-?)}{Man(2-?)}Man(?)[Man(?)]Man(?)[Man(?... |
Man6 | cis_golgi | {Man(1-?)}Man(?)[Man(?)]Man(?)[Man(?)]Man(?)Gl... |
Man5 | cis_golgi | Man(?)[Man(?)]Man(?)[Man(?)]Man(?)GlcNAc(?)GlcNAc |
G0-GlcNAc | medial_golgi | Man(?)[GlcNAc(?)Man(?)]Man(?)GlcNAc(?)GlcNAc |
G0 | medial_golgi | GlcNAc(?)Man(?)[GlcNAc(?)Man(?)]Man(?)GlcNAc(?... |
G0F | medial_golgi | GlcNAc(?)Man(?)[GlcNAc(?)Man(?)]Man(?)GlcNAc(?... |
G0F-GlcNAc | medial_golgi | Man(?)[GlcNAc(?)Man(?)]Man(?)GlcNAc(?)[Fuc(?)]... |
G0F+GlcNAc | medial_golgi | GlcNAc(?)[GlcNAc(?)]Man(?)[GlcNAc(?)Man(?)]Man... |
G1a/b | trans Golgi | {Gal(1-?)}GlcNAc(?)Man(?)[GlcNAc(?)Man(?)]Man(... |
G1Fa/b | trans Golgi | {Gal(1-?)}GlcNAc(?)Man(?)[GlcNAc(?)Man(?)]Man(... |
G2F | trans Golgi | Gal(?)GlcNAc(?)Man(?)[Gal(?)GlcNAc(?)Man(?)]Ma... |
A1G1F | trans Golgi | {Neu5Ac(1-?)Gal()}GlcNAc(?)Man(?)[GlcNAc(?)Man... |
A1G2F | trans Golgi | {Neu5Ac(1-?)}Gal(?)GlcNAc(?)Man(?)[Gal(?)GlcNA... |
Reaction Description | Enzymes | Enzyme Name | Notes | Genes | |
---|---|---|---|---|---|
Reaction ID | |||||
Ent_Man7 | Entry of Man7 | NaN | NaN | NaN | NaN |
Ex_Man7 | Secretion of Man7 | NaN | NaN | NaN | NaN |
Ex_Man6 | Secretion of Man6 | NaN | NaN | NaN | NaN |
Ex_Man5 | Secretion of Man5 | NaN | NaN | NaN | NaN |
Ex_G0-GlcNAc | Secretion of G0-GlcNAc | NaN | NaN | NaN | NaN |
Ex_G0 | Secretion of G0 | NaN | NaN | NaN | NaN |
Ex_G0F | Secretion of G0F | NaN | NaN | NaN | NaN |
Ex_G0F-GlcNAc | Secretion of G0F-GlcNAc | NaN | NaN | NaN | NaN |
Ex_G0F+GlcNAc | Secretion of G0F+GlcNAc | NaN | NaN | NaN | NaN |
Ex_G1a/b | Secretion of G1a/b | NaN | NaN | NaN | NaN |
Ex_G1Fa/b | Secretion of G1Fa/b | NaN | NaN | NaN | NaN |
Ex_G2F | Secretion of G2F | NaN | NaN | NaN | NaN |
Ex_A1G1F | Secretion of A1G1F | NaN | NaN | NaN | NaN |
Ex_A1G2F | Secretion of A1G2F | NaN | NaN | NaN | NaN |
R1 | Man7 trimmed to Man6 | ManI | Mannosidase | NaN | MAN1A1, MAN1A2 |
R2 | Man 6 trimmed to Man5 | ManI | Mannosidase | NaN | MAN1A1, MAN1A2 |
R3 | Man5 undergoes trimming and GlcNAc addition to... | GnTI/ManII | Multiple enzymes | MGAT1 followed by MAN2A1/MAN2A2 | MGAT1, MAN2A1, MAN2A2 |
R4 | GlcNAc addition to form G0 | GnTII | Glucosaminyltransferase | NaN | MGAT2 |
R5 | GlcNAc addition to form G0F | GnTII | Glucosaminyltransferase | NaN | MGAT2 |
R8 | GlcNAc addition to form G0F+GlcNAc | GnTIV | Glucosaminyltransferase | NaN | MGAT4A, MGAT4B, MGAT5 |
R6 | Fucosylation of N-glycans | FucT | Fucosyltransferase | FUT10, FUT11 in invertebrates/plants | FUT8, FUT10, FUT11 |
R7 | Fucosylation of N-glycans | FucT | Fucosyltransferase | FUT10, FUT11 in invertebrates/plants | FUT8, FUT10, FUT11 |
R9 | Fucosylation of N-glycans | FucT | Fucosyltransferase | FUT10, FUT11 in invertebrates/plants | FUT8, FUT10, FUT11 |
R10 | Galactose addition | GalT | Galactosyltransferase | NaN | B4GALT1, B4GALT2, B4GALT3, B4GALT4, B4GALT5, B... |
R11 | Galactose addition | GalT | Galactosyltransferase | NaN | B4GALT1, B4GALT2, B4GALT3, B4GALT4, B4GALT5, B... |
R12 | Galactose addition | GalT | Galactosyltransferase | NaN | B4GALT1, B4GALT2, B4GALT3, B4GALT4, B4GALT5, B... |
R13 | Galactose addition | GalT | Galactosyltransferase | NaN | B4GALT1, B4GALT2, B4GALT3, B4GALT4, B4GALT5, B... |
R14 | Neu5Ac addition | SiaT | Sialyltransferase | NaN | ST3GAL3, ST3GAL4, ST3GAL6 |
R15 | Neu5Ac addition | SiaT | Sialyltransferase | NaN | ST3GAL3, ST3GAL4, ST3GAL6 |
Read and format data¶
pH_scale = 'highpH'
expt_data = pd.read_excel(os.path.join(inputDir, 'Lee et al', pH_scale+'_processed.xlsx'), sheet_name=None, index_col=0)
expt_data['q_prod'].loc[13, :] = expt_data['q_prod'].loc[12, :]
expt_data['q_prod'] = expt_data['q_prod'].drop(index=[12, 14])
expt_data['Fractions'].loc[13, :] = expt_data['Fractions'].loc[[12, 14], :].mean()
expt_data['Fractions'] = expt_data['Fractions'].drop(index=[12, 14])
expt_data['fit_Fractions'] = expt_data['fit_Concentrations'].div(expt_data['Titer']['Titer (g/L)'], axis=0)
Comments: Multiply titer with 1000 for unit consistency $$ v_{E, i}(t) = \frac{1}{VCD(t)} \cdot \frac{dfrac_{i}(t) \cdot C_{mAb}(t)}{dt} + frac_{i}(t) \cdot \left (Q_{prod}(t) - \frac{dC_{mAB}(t)}{dt} \cdot \frac{1}{VCD}\right)\ $$
dtiter_VCD = (expt_data['Titer']['diff_Titer (g/L)'].div(expt_data['VCD']['VCD (1E6 VC/mL)']))*1000
secondterm = (expt_data['Fractions'].
mul(expt_data['q_prod']['Spec Prod (pg/cells/day)'] - dtiter_VCD[expt_data['q_prod'].index], axis=0).
dropna())
dC_VCD = (expt_data['diff_Concentrations'].div(expt_data['VCD']['VCD (1E6 VC/mL)'], axis=0).dropna())*1000
expt_data['Secreted Flux'] = dC_VCD.loc[expt_data['q_prod'].index, :] + secondterm
display(expt_data.keys())
dict_keys(['VCD', 'Titer', 'Fractions', 'q_prod', 'Concentrations', 'fit_Concentrations', 'diff_Concentrations', 'fit_Fractions', 'Secreted Flux'])
fig, ax = plt.subplots(1, 3, figsize=(15, 5))
sns.barplot(data=expt_data['q_prod'], x='Time window (days)', y='Spec Prod (pg/cells/day)', ax=ax[0], facecolor='#F7F6F6', edgecolor='k', hatch='/', fill=True)
ax[0].set_title('Specific Productivity')
plot_fig(time_col='Time (WD)', data=expt_data['Titer'], meas_col='Titer (g/L)', smooth_col='fit_Titer (g/L)', ax=ax[1])
ax[1].set_title('Titer')
plot_fig(time_col='Time (WD)', data=expt_data['VCD'], meas_col='VCD (1E6 VC/mL)', smooth_col='fit_VCD (1E6 VC/mL)', ax=ax[2])
ax[2].set_title('VCD')
plt.show()
/home/cabsel/miniconda3/envs/Shri/lib/python3.11/site-packages/matplotlib/axes/_axes.py:1185: RuntimeWarning: All-NaN axis encountered
miny = np.nanmin(masked_verts[..., 1])
/home/cabsel/miniconda3/envs/Shri/lib/python3.11/site-packages/matplotlib/axes/_axes.py:1186: RuntimeWarning: All-NaN axis encountered
maxy = np.nanmax(masked_verts[..., 1])
/home/cabsel/miniconda3/envs/Shri/lib/python3.11/site-packages/matplotlib/axes/_axes.py:1185: RuntimeWarning: All-NaN axis encountered
miny = np.nanmin(masked_verts[..., 1])
/home/cabsel/miniconda3/envs/Shri/lib/python3.11/site-packages/matplotlib/axes/_axes.py:1186: RuntimeWarning: All-NaN axis encountered
maxy = np.nanmax(masked_verts[..., 1])
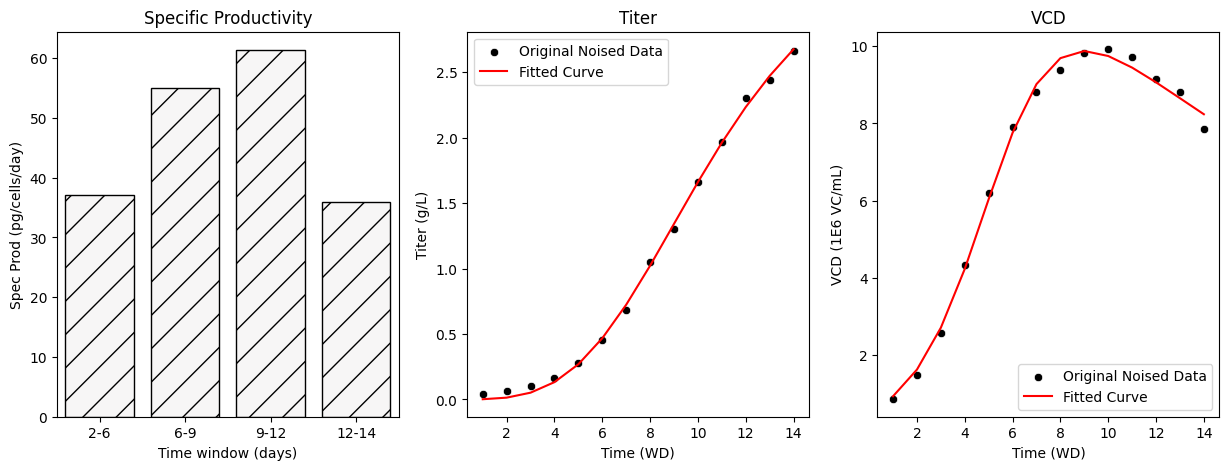
Initialize iGFA and ingest experimental measurements into iGFA’s class¶
linear_rxns = ['R1', 'R2', 'R3'] # 'R1', 'R2', 'R3', 'R4', 'R5', 'R8', 'R6', 'R7', 'R9', 'R10', 'R11', 'R12', 'R13', 'R14', 'R15'
init_model = parse_model(stoich_data, rxn_meta, met_meta, 1, linear_rxns)
init_model.read_measurements(expt_data, time_col_name='Time (WD)')
data = init_model.fetch_data(spec_prod_col='Spec Prod (pg/cells/day)')
# Use get_diff=True only when derivative of fractions is precalculated
print(data[None].keys())
dict_keys(['timepoints', 'timepoints_data', 'spec_prod', 'secreted_flux_data'])
data[None]['reg_param'] = {None: 100}
model = init_model.create_pyomomodel(fit_beta=True, regularize_params=True) # Use precalc_diff only when derivative of fractions is precalculated
instance = model.create_instance(data=data)
# discretizer = pyo.TransformationFactory('dae.finite_difference')
# discretizer.apply_to(instance, nfe=3, wrt=model.timepoints, scheme='CENTRAL')
summarize_instance(instance)
number_of_constraints: 53
number_of_objectives: 1
number_of_variables: 84
Names of variables in model:
entry_flux : 4 variables
lin_flux : 12 variables
v_ref : 12 variables
alpha : 20 variables
beta : 32 variables
gamma : 4 variables
Names of constraints in model:
pos_secretions : 52 constraints
min_params : 1 constraints
Names of objectives in model:
obj : 1 objective
timepoints : Size=1, Index=None, Ordered=Sorted
Key : Dimen : Domain : Size : Members
None : 1 : Any : 4 : {5, 8, 10, 13}
None
mets : Size=1, Index=None, Ordered=Insertion
Key : Dimen : Domain : Size : Members
None : 1 : Any : 13 : {'Man7', 'Man6', 'Man5', 'G0-GlcNAc', 'G0', 'G0F', 'G0F-GlcNAc', 'G0F+GlcNAc', 'G1a/b', 'G1Fa/b', 'G2F', 'A1G1F', 'A1G2F'}
None
enzymes : Size=1, Index=None, Ordered=Insertion
Key : Dimen : Domain : Size : Members
None : 1 : Any : 5 : {'FucT', 'GalT', 'GnTII', 'GnTIV', 'SiaT'}
None
internal_rxns : Size=1, Index=None, Ordered=Insertion
Key : Dimen : Domain : Size : Members
None : 1 : Any : 15 : {'R1', 'R2', 'R3', 'R4', 'R5', 'R8', 'R6', 'R7', 'R9', 'R10', 'R11', 'R12', 'R13', 'R14', 'R15'}
None
Single start¶
# result = init_model.run_singlestart(instance)
# solver = pyo.SolverFactory('ipopt')
# compute_infeasibility_explanation(instance, solver)
# pyo.value(instance.alpha[5, 'FucT'])
Multi start¶
results_dict, status_run = init_model.run_multistart(instance, strategy='rand_guess_and_bound', iterations=100, suppress_warning=True,
solver_options={'print_level': 4}) # 'tol': 1e-5, 'constr_viol_tol': 1e-5
Show code cell output
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 2944
(scaled) (unscaled)
Objective...............: 1.2713811175031994e+02 1.2713811175031994e+02
Dual infeasibility......: 1.0045770297741345e+04 1.0045770297741345e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 5.4383436271719260e-09 5.4383436271719260e-09
Overall NLP error.......: 1.0045770297741345e+04 1.0045770297741345e+04
Number of objective function evaluations = 3324
Number of objective gradient evaluations = 2927
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 3325
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 2948
Number of Lagrangian Hessian evaluations = 2945
Total seconds in IPOPT = 2.883
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 2.9024105072021484
Solution:
- number of solutions: 0
number of solutions displayed: 0
0 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 287
(scaled) (unscaled)
Objective...............: 5.2650053535286864e-06 3.0586169934289501e+02
Dual infeasibility......: 6.1822580109221386e-04 3.5914796168812070e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 5.8043850000271039e-09 3.3719605974547406e-01
Overall NLP error.......: 6.1822580109221386e-04 3.5914796168812070e+04
Number of objective function evaluations = 490
Number of objective gradient evaluations = 266
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 491
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 290
Number of Lagrangian Hessian evaluations = 288
Total seconds in IPOPT = 0.299
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.3244059085845947
Solution:
- number of solutions: 0
number of solutions displayed: 0
1 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 295
(scaled) (unscaled)
Objective...............: 5.0227263766094073e-06 5.0227263766094075e+02
Dual infeasibility......: 4.7427266691903603e-04 4.7427266691903606e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.6218446681496190e-08 2.6218446681496190e+00
Overall NLP error.......: 4.7427266691903603e-04 4.7427266691903606e+04
Number of objective function evaluations = 617
Number of objective gradient evaluations = 284
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 617
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 298
Number of Lagrangian Hessian evaluations = 296
Total seconds in IPOPT = 0.316
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.3396930694580078
Solution:
- number of solutions: 0
number of solutions displayed: 0
2 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 352
(scaled) (unscaled)
Objective...............: 2.7431826692052686e-09 2.7431826692052685e-01
Dual infeasibility......: 1.4891825055003255e-09 1.0157870832573417e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544316382654648e-13 1.2544316382654647e-05
Overall NLP error.......: 1.4891825055003255e-09 1.0157870832573417e-01
Number of objective function evaluations = 827
Number of objective gradient evaluations = 353
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 827
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 353
Number of Lagrangian Hessian evaluations = 352
Total seconds in IPOPT = 0.384
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4102442264556885
Solution:
- number of solutions: 0
number of solutions displayed: 0
3 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 265
(scaled) (unscaled)
Objective...............: 3.9533848682690785e-06 3.9533848682690785e+02
Dual infeasibility......: 4.3931300235687038e-04 4.3931300235687035e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3337614452399724e-08 1.3337614452399724e+00
Overall NLP error.......: 4.3931300235687038e-04 4.3931300235687035e+04
Number of objective function evaluations = 392
Number of objective gradient evaluations = 246
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 392
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 268
Number of Lagrangian Hessian evaluations = 266
Total seconds in IPOPT = 0.278
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.30240654945373535
Solution:
- number of solutions: 0
number of solutions displayed: 0
4 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 410
(scaled) (unscaled)
Objective...............: 2.1879893892098237e-09 2.1879893892098237e-01
Dual infeasibility......: 1.3191538075597193e-09 1.3191538075597192e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2605007239071396e-13 1.2605007239071397e-05
Overall NLP error.......: 1.3191538075597193e-09 1.3191538075597192e-01
Number of objective function evaluations = 1086
Number of objective gradient evaluations = 411
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1086
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 411
Number of Lagrangian Hessian evaluations = 410
Total seconds in IPOPT = 0.453
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4784533977508545
Solution:
- number of solutions: 0
number of solutions displayed: 0
5 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 346
(scaled) (unscaled)
Objective...............: 2.7908617507694593e-09 2.7908617507694594e-01
Dual infeasibility......: 3.9531713162050397e-09 3.9531713162050397e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544306559264640e-13 1.2544306559264639e-05
Overall NLP error.......: 3.9531713162050397e-09 3.9531713162050397e-01
Number of objective function evaluations = 913
Number of objective gradient evaluations = 347
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 913
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 347
Number of Lagrangian Hessian evaluations = 346
Total seconds in IPOPT = 0.380
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4054388999938965
Solution:
- number of solutions: 0
number of solutions displayed: 0
6 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 449
(scaled) (unscaled)
Objective...............: 3.0575178846231671e-09 3.0575178846231671e-01
Dual infeasibility......: 7.0737257025011241e-09 7.0737257025011246e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544396082305815e-13 1.2544396082305814e-05
Overall NLP error.......: 7.0737257025011241e-09 7.0737257025011246e-01
Number of objective function evaluations = 1923
Number of objective gradient evaluations = 450
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1924
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 451
Number of Lagrangian Hessian evaluations = 449
Total seconds in IPOPT = 0.502
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5270059108734131
Solution:
- number of solutions: 0
number of solutions displayed: 0
7 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 291
(scaled) (unscaled)
Objective...............: 3.6425808392227948e-09 3.6425808392227949e-01
Dual infeasibility......: 9.6216743142754520e-09 4.7162400338321114e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 4.0847371771990124e-13 4.0847371771990123e-05
Overall NLP error.......: 9.6216743142754520e-09 4.7162400338321114e-01
Number of objective function evaluations = 468
Number of objective gradient evaluations = 292
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 468
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 292
Number of Lagrangian Hessian evaluations = 291
Total seconds in IPOPT = 0.306
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3310372829437256
Solution:
- number of solutions: 0
number of solutions displayed: 0
8 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 353
(scaled) (unscaled)
Objective...............: 5.1033789832574040e-09 5.1033789832574039e-01
Dual infeasibility......: 1.4858879859329830e-09 1.4858879859329829e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 7.9023617131321722e-13 7.9023617131321718e-05
Overall NLP error.......: 1.4858879859329830e-09 1.4858879859329829e-01
Number of objective function evaluations = 582
Number of objective gradient evaluations = 354
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 582
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 354
Number of Lagrangian Hessian evaluations = 353
Total seconds in IPOPT = 0.383
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.40851521492004395
Solution:
- number of solutions: 0
number of solutions displayed: 0
9 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 307
(scaled) (unscaled)
Objective...............: 3.6138805452850772e-09 3.6138805452850770e-01
Dual infeasibility......: 6.9786860750507428e-07 6.9786860750507429e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 8.3769843258257046e-13 8.3769843258257049e-05
Overall NLP error.......: 6.9786860750507428e-07 6.9786860750507429e+01
Number of objective function evaluations = 609
Number of objective gradient evaluations = 309
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 609
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 309
Number of Lagrangian Hessian evaluations = 308
Total seconds in IPOPT = 0.345
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.36908507347106934
Solution:
- number of solutions: 0
number of solutions displayed: 0
10 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 373
(scaled) (unscaled)
Objective...............: 7.1106613208543808e-06 7.1106613208543808e+02
Dual infeasibility......: 6.0958111751187349e-04 6.0958111751187345e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 4.9037851087980913e-09 4.9037851087980910e-01
Overall NLP error.......: 6.0958111751187349e-04 6.0958111751187345e+04
Number of objective function evaluations = 688
Number of objective gradient evaluations = 353
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 689
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 376
Number of Lagrangian Hessian evaluations = 374
Total seconds in IPOPT = 0.399
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.4234802722930908
Solution:
- number of solutions: 0
number of solutions displayed: 0
11 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 296
(scaled) (unscaled)
Objective...............: 3.0990701630670116e-09 3.0990701630670114e-01
Dual infeasibility......: 9.6655335671587559e-07 9.6655335671587565e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5523752512431530e-13 1.5523752512431529e-05
Overall NLP error.......: 9.6655335671587559e-07 9.6655335671587565e+01
Number of objective function evaluations = 619
Number of objective gradient evaluations = 288
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 619
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 299
Number of Lagrangian Hessian evaluations = 297
Total seconds in IPOPT = 0.328
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.351886510848999
Solution:
- number of solutions: 0
number of solutions displayed: 0
12 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 280
(scaled) (unscaled)
Objective...............: 5.5986557886632577e-06 5.5986557886632579e+02
Dual infeasibility......: 5.1408356541331017e-04 5.1408356541331013e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.6859629790478345e-09 3.6859629790478343e-01
Overall NLP error.......: 5.1408356541331017e-04 5.1408356541331013e+04
Number of objective function evaluations = 563
Number of objective gradient evaluations = 270
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 564
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 283
Number of Lagrangian Hessian evaluations = 281
Total seconds in IPOPT = 0.311
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.3351585865020752
Solution:
- number of solutions: 0
number of solutions displayed: 0
13 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 328
(scaled) (unscaled)
Objective...............: 3.6243384385804145e-09 3.6243384385804145e-01
Dual infeasibility......: 6.4547147008762520e-07 6.4547147008762522e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 9.9999980657239362e-09 9.9999980657239362e-09
Complementarity.........: 1.3003113172491350e-12 1.3003113172491349e-04
Overall NLP error.......: 6.4547147008762520e-07 6.4547147008762522e+01
Number of objective function evaluations = 531
Number of objective gradient evaluations = 330
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 531
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 330
Number of Lagrangian Hessian evaluations = 329
Total seconds in IPOPT = 0.346
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.3698244094848633
Solution:
- number of solutions: 0
number of solutions displayed: 0
14 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 371
(scaled) (unscaled)
Objective...............: 2.6393729766584647e-09 2.6393729766584645e-01
Dual infeasibility......: 6.4593038201356525e-09 6.4593038201356523e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544337250308721e-13 1.2544337250308721e-05
Overall NLP error.......: 6.4593038201356525e-09 6.4593038201356523e-01
Number of objective function evaluations = 548
Number of objective gradient evaluations = 372
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 549
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 372
Number of Lagrangian Hessian evaluations = 371
Total seconds in IPOPT = 0.395
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.41905856132507324
Solution:
- number of solutions: 0
number of solutions displayed: 0
15 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 371
(scaled) (unscaled)
Objective...............: 1.8435383422065017e-09 1.8435383422065016e-01
Dual infeasibility......: 8.3026006872551536e-09 8.3026006872551539e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544302826334679e-13 1.2544302826334679e-05
Overall NLP error.......: 8.3026006872551536e-09 8.3026006872551539e-01
Number of objective function evaluations = 573
Number of objective gradient evaluations = 372
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 573
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 373
Number of Lagrangian Hessian evaluations = 371
Total seconds in IPOPT = 0.395
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4187967777252197
Solution:
- number of solutions: 0
number of solutions displayed: 0
16 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 290
(scaled) (unscaled)
Objective...............: 3.2027743979163269e-09 3.2027743979163270e-01
Dual infeasibility......: 7.2784886514381260e-07 7.2784886514381256e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 8.8292724901202519e-12 8.8292724901202512e-04
Overall NLP error.......: 7.2784886514381260e-07 7.2784886514381256e+01
Number of objective function evaluations = 629
Number of objective gradient evaluations = 292
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 630
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 293
Number of Lagrangian Hessian evaluations = 291
Total seconds in IPOPT = 0.317
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.34097862243652344
Solution:
- number of solutions: 0
number of solutions displayed: 0
17 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 459
(scaled) (unscaled)
Objective...............: 4.9941630648654975e-09 4.9941630648654972e-01
Dual infeasibility......: 1.3557934265401388e-07 1.3557934265401387e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544302826334684e-13 1.2544302826334684e-05
Overall NLP error.......: 1.3557934265401388e-07 1.3557934265401387e+01
Number of objective function evaluations = 2150
Number of objective gradient evaluations = 461
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 2150
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 461
Number of Lagrangian Hessian evaluations = 460
Total seconds in IPOPT = 0.503
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.5276548862457275
Solution:
- number of solutions: 0
number of solutions displayed: 0
18 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 401
(scaled) (unscaled)
Objective...............: 3.5138804573124354e-09 3.5138804573124355e-01
Dual infeasibility......: 8.3160532383734945e-09 8.3160532383734942e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2596101008840648e-13 1.2596101008840648e-05
Overall NLP error.......: 8.3160532383734945e-09 8.3160532383734942e-01
Number of objective function evaluations = 965
Number of objective gradient evaluations = 402
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 965
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 402
Number of Lagrangian Hessian evaluations = 401
Total seconds in IPOPT = 0.458
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4817211627960205
Solution:
- number of solutions: 0
number of solutions displayed: 0
19 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 390
(scaled) (unscaled)
Objective...............: 3.9605255684521686e-09 3.9605255684521684e-01
Dual infeasibility......: 2.1206922757114766e-09 2.1206922757114766e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3903305158800546e-13 1.3903305158800546e-05
Overall NLP error.......: 2.1206922757114766e-09 2.1206922757114766e-01
Number of objective function evaluations = 899
Number of objective gradient evaluations = 391
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 899
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 391
Number of Lagrangian Hessian evaluations = 390
Total seconds in IPOPT = 0.423
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.44733619689941406
Solution:
- number of solutions: 0
number of solutions displayed: 0
20 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 377
(scaled) (unscaled)
Objective...............: 3.3960689355614626e-09 3.3960689355614626e-01
Dual infeasibility......: 8.9416131239172564e-07 8.9416131239172557e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6256275801000504e-13 1.6256275801000505e-05
Overall NLP error.......: 8.9416131239172564e-07 8.9416131239172557e+01
Number of objective function evaluations = 597
Number of objective gradient evaluations = 379
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 597
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 379
Number of Lagrangian Hessian evaluations = 378
Total seconds in IPOPT = 0.400
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.4251258373260498
Solution:
- number of solutions: 0
number of solutions displayed: 0
21 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 921
(scaled) (unscaled)
Objective...............: 3.5510229574803747e-09 3.5510229574803748e-01
Dual infeasibility......: 9.6514435191186956e-09 9.6514435191186954e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2545073145974100e-13 1.2545073145974100e-05
Overall NLP error.......: 9.6514435191186956e-09 9.6514435191186954e-01
Number of objective function evaluations = 7756
Number of objective gradient evaluations = 907
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 7756
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 923
Number of Lagrangian Hessian evaluations = 921
Total seconds in IPOPT = 1.065
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 1.0885608196258545
Solution:
- number of solutions: 0
number of solutions displayed: 0
22 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 384
(scaled) (unscaled)
Objective...............: 3.9374033997927626e-09 3.9374033997927627e-01
Dual infeasibility......: 5.7730672500086214e-09 5.7730672500086211e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3008240769365323e-13 1.3008240769365323e-05
Overall NLP error.......: 5.7730672500086214e-09 5.7730672500086211e-01
Number of objective function evaluations = 659
Number of objective gradient evaluations = 385
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 660
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 385
Number of Lagrangian Hessian evaluations = 384
Total seconds in IPOPT = 0.420
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.44492673873901367
Solution:
- number of solutions: 0
number of solutions displayed: 0
23 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 385
(scaled) (unscaled)
Objective...............: 2.0672263790486895e-09 2.0672263790486894e-01
Dual infeasibility......: 3.7491773555861207e-09 3.7491773555861208e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.1890712240318613e-13 3.1890712240318611e-05
Overall NLP error.......: 3.7491773555861207e-09 3.7491773555861208e-01
Number of objective function evaluations = 635
Number of objective gradient evaluations = 386
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 635
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 386
Number of Lagrangian Hessian evaluations = 385
Total seconds in IPOPT = 0.412
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4375729560852051
Solution:
- number of solutions: 0
number of solutions displayed: 0
24 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 361
(scaled) (unscaled)
Objective...............: 1.9419721215204026e-09 1.9419721215204025e-01
Dual infeasibility......: 3.7929521925070730e-09 3.7929521925070731e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2546966081174906e-13 1.2546966081174905e-05
Overall NLP error.......: 3.7929521925070730e-09 3.7929521925070731e-01
Number of objective function evaluations = 549
Number of objective gradient evaluations = 362
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 550
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 362
Number of Lagrangian Hessian evaluations = 361
Total seconds in IPOPT = 0.391
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4166140556335449
Solution:
- number of solutions: 0
number of solutions displayed: 0
25 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 303
(scaled) (unscaled)
Objective...............: 3.1899150152135238e-09 3.1899150152135236e-01
Dual infeasibility......: 9.1414124910455082e-09 9.1414124910455086e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.1739052635366779e-13 2.1739052635366778e-05
Overall NLP error.......: 9.1414124910455082e-09 9.1414124910455086e-01
Number of objective function evaluations = 639
Number of objective gradient evaluations = 303
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 640
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 305
Number of Lagrangian Hessian evaluations = 303
Total seconds in IPOPT = 0.330
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3536694049835205
Solution:
- number of solutions: 0
number of solutions displayed: 0
26 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 370
(scaled) (unscaled)
Objective...............: 5.8935518066776353e-06 5.8935518066776353e+02
Dual infeasibility......: 5.1501041334090219e-04 5.1501041334090216e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.8585003872951646e-09 3.8585003872951645e-01
Overall NLP error.......: 5.1501041334090219e-04 5.1501041334090216e+04
Number of objective function evaluations = 905
Number of objective gradient evaluations = 365
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 906
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 373
Number of Lagrangian Hessian evaluations = 371
Total seconds in IPOPT = 0.410
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.43409228324890137
Solution:
- number of solutions: 0
number of solutions displayed: 0
27 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 292
(scaled) (unscaled)
Objective...............: 3.5121322725464701e-09 3.5121322725464699e-01
Dual infeasibility......: 2.2702395169388500e-09 2.2702395169388501e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3051904024718246e-13 1.3051904024718246e-05
Overall NLP error.......: 2.2702395169388500e-09 2.2702395169388501e-01
Number of objective function evaluations = 445
Number of objective gradient evaluations = 293
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 445
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 293
Number of Lagrangian Hessian evaluations = 292
Total seconds in IPOPT = 0.320
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3434138298034668
Solution:
- number of solutions: 0
number of solutions displayed: 0
28 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 1215
(scaled) (unscaled)
Objective...............: 5.7010512384573350e-06 5.7010512384573349e+02
Dual infeasibility......: 5.3062655013344138e-04 5.3062655013344134e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.7342602890661164e-08 2.7342602890661163e+00
Overall NLP error.......: 5.3062655013344138e-04 5.3062655013344134e+04
Number of objective function evaluations = 10858
Number of objective gradient evaluations = 1208
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 10859
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 1218
Number of Lagrangian Hessian evaluations = 1216
Total seconds in IPOPT = 1.318
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 1.3416152000427246
Solution:
- number of solutions: 0
number of solutions displayed: 0
29 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 382
(scaled) (unscaled)
Objective...............: 3.0497395620761509e-09 3.0497395620761508e-01
Dual infeasibility......: 2.3931123077381536e-09 2.3931123077381536e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6385812933921625e-13 1.6385812933921625e-05
Overall NLP error.......: 2.3931123077381536e-09 2.3931123077381536e-01
Number of objective function evaluations = 697
Number of objective gradient evaluations = 383
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 697
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 383
Number of Lagrangian Hessian evaluations = 382
Total seconds in IPOPT = 0.424
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4490387439727783
Solution:
- number of solutions: 0
number of solutions displayed: 0
30 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 313
(scaled) (unscaled)
Objective...............: 2.7877529107514295e-06 2.7877529107514295e+02
Dual infeasibility......: 3.3454815336870348e-04 3.3454815336870350e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 5.5036502385521464e-09 5.5036502385521469e-01
Overall NLP error.......: 3.3454815336870348e-04 3.3454815336870350e+04
Number of objective function evaluations = 483
Number of objective gradient evaluations = 302
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 484
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 316
Number of Lagrangian Hessian evaluations = 314
Total seconds in IPOPT = 0.339
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.36483144760131836
Solution:
- number of solutions: 0
number of solutions displayed: 0
31 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 503
(scaled) (unscaled)
Objective...............: 3.4355291051013256e-09 3.4355291051013254e-01
Dual infeasibility......: 4.4243315432937583e-09 3.2047494193906378e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2690071885260718e-13 1.2690071885260718e-05
Overall NLP error.......: 4.4243315432937583e-09 3.2047494193906378e-01
Number of objective function evaluations = 2749
Number of objective gradient evaluations = 504
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 2749
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 504
Number of Lagrangian Hessian evaluations = 503
Total seconds in IPOPT = 0.568
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5921304225921631
Solution:
- number of solutions: 0
number of solutions displayed: 0
32 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 296
(scaled) (unscaled)
Objective...............: 3.0195600100594301e-09 3.0195600100594300e-01
Dual infeasibility......: 2.1288521727270748e-07 2.1288521727270748e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5950911713999592e-13 1.5950911713999593e-05
Overall NLP error.......: 2.1288521727270748e-07 2.1288521727270748e+01
Number of objective function evaluations = 507
Number of objective gradient evaluations = 297
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 507
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 297
Number of Lagrangian Hessian evaluations = 296
Total seconds in IPOPT = 0.320
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.34439659118652344
Solution:
- number of solutions: 0
number of solutions displayed: 0
33 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 391
(scaled) (unscaled)
Objective...............: 1.9105962591997883e-09 1.9105962591997883e-01
Dual infeasibility......: 5.5360296650552333e-09 5.5360296650552332e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544320683158759e-13 1.2544320683158759e-05
Overall NLP error.......: 5.5360296650552333e-09 5.5360296650552332e-01
Number of objective function evaluations = 616
Number of objective gradient evaluations = 392
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 617
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 392
Number of Lagrangian Hessian evaluations = 391
Total seconds in IPOPT = 0.418
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4414215087890625
Solution:
- number of solutions: 0
number of solutions displayed: 0
34 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 340
(scaled) (unscaled)
Objective...............: 3.3242595560362952e-09 3.3242595560362953e-01
Dual infeasibility......: 2.0630898842231090e-09 1.7996221908019952e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2838938268862243e-13 1.2838938268862243e-05
Overall NLP error.......: 2.0630898842231090e-09 1.7996221908019952e-01
Number of objective function evaluations = 733
Number of objective gradient evaluations = 341
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 733
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 341
Number of Lagrangian Hessian evaluations = 340
Total seconds in IPOPT = 0.373
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3966550827026367
Solution:
- number of solutions: 0
number of solutions displayed: 0
35 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 201
(scaled) (unscaled)
Objective...............: 1.3733515390779373e-05 1.3733515390779373e+03
Dual infeasibility......: 1.7460732658733220e-03 1.7460732658733221e+05
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.9131994012191564e-08 1.9131994012191564e+00
Overall NLP error.......: 1.7460732658733220e-03 1.7460732658733221e+05
Number of objective function evaluations = 295
Number of objective gradient evaluations = 183
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 295
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 204
Number of Lagrangian Hessian evaluations = 202
Total seconds in IPOPT = 0.215
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.23835039138793945
Solution:
- number of solutions: 0
number of solutions displayed: 0
36 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 387
(scaled) (unscaled)
Objective...............: 2.9302841383800178e-09 2.7293578084797998e-01
Dual infeasibility......: 1.8617513038065635e-09 1.7340930839905142e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3720260687803244e-13 1.2779477645924377e-05
Overall NLP error.......: 1.8617513038065635e-09 1.7340930839905142e-01
Number of objective function evaluations = 710
Number of objective gradient evaluations = 378
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 710
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 389
Number of Lagrangian Hessian evaluations = 387
Total seconds in IPOPT = 0.416
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.43959736824035645
Solution:
- number of solutions: 0
number of solutions displayed: 0
37 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 550
(scaled) (unscaled)
Objective...............: 3.5201450707024333e-09 3.5201450707024334e-01
Dual infeasibility......: 2.1127122845863191e-09 2.1127122845863192e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2556172573156121e-13 1.2556172573156121e-05
Overall NLP error.......: 2.1127122845863191e-09 2.1127122845863192e-01
Number of objective function evaluations = 1407
Number of objective gradient evaluations = 546
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1408
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 552
Number of Lagrangian Hessian evaluations = 550
Total seconds in IPOPT = 0.616
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.6360342502593994
Solution:
- number of solutions: 0
number of solutions displayed: 0
38 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 305
(scaled) (unscaled)
Objective...............: 3.4602150802361211e-09 3.4602150802361209e-01
Dual infeasibility......: 2.6272479737190633e-07 2.6272479737190633e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5693124336028712e-13 1.5693124336028710e-05
Overall NLP error.......: 2.6272479737190633e-07 2.6272479737190633e+01
Number of objective function evaluations = 538
Number of objective gradient evaluations = 307
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 539
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 308
Number of Lagrangian Hessian evaluations = 306
Total seconds in IPOPT = 0.329
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.35202646255493164
Solution:
- number of solutions: 0
number of solutions displayed: 0
39 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 349
(scaled) (unscaled)
Objective...............: 2.8121290472327995e-06 2.8121290472327996e+02
Dual infeasibility......: 3.7358271680064544e-04 3.7358271680064543e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5259801007905830e-09 1.5259801007905829e-01
Overall NLP error.......: 3.7358271680064544e-04 3.7358271680064543e+04
Number of objective function evaluations = 628
Number of objective gradient evaluations = 325
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 628
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 353
Number of Lagrangian Hessian evaluations = 350
Total seconds in IPOPT = 0.373
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.39913487434387207
Solution:
- number of solutions: 0
number of solutions displayed: 0
40 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 291
(scaled) (unscaled)
Objective...............: 7.5784005239925444e-09 7.5784005239925445e-01
Dual infeasibility......: 3.5623804485075565e-09 3.5623804485075566e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 8.0929677525408819e-13 8.0929677525408821e-05
Overall NLP error.......: 3.5623804485075565e-09 3.5623804485075566e-01
Number of objective function evaluations = 606
Number of objective gradient evaluations = 292
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 607
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 292
Number of Lagrangian Hessian evaluations = 291
Total seconds in IPOPT = 0.323
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.34604644775390625
Solution:
- number of solutions: 0
number of solutions displayed: 0
41 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 300
(scaled) (unscaled)
Objective...............: 3.9627712874780683e-09 3.9627712874780679e-01
Dual infeasibility......: 2.2413914189586892e-09 2.2413914189586892e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4022798537550022e-13 1.4022798537550021e-05
Overall NLP error.......: 2.2413914189586892e-09 2.2413914189586892e-01
Number of objective function evaluations = 552
Number of objective gradient evaluations = 301
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 553
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 301
Number of Lagrangian Hessian evaluations = 300
Total seconds in IPOPT = 0.327
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3507668972015381
Solution:
- number of solutions: 0
number of solutions displayed: 0
42 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 384
(scaled) (unscaled)
Objective...............: 2.8932782216764958e-09 2.8932782216764957e-01
Dual infeasibility......: 1.3162353979970675e-09 9.2903603594978915e-02
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2689129854904841e-13 1.2689129854904841e-05
Overall NLP error.......: 1.3162353979970675e-09 9.2903603594978915e-02
Number of objective function evaluations = 824
Number of objective gradient evaluations = 385
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 825
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 385
Number of Lagrangian Hessian evaluations = 384
Total seconds in IPOPT = 0.412
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4367098808288574
Solution:
- number of solutions: 0
number of solutions displayed: 0
43 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 320
(scaled) (unscaled)
Objective...............: 3.1465357636821983e-06 3.1465357636821983e+02
Dual infeasibility......: 3.6330139898326511e-04 3.6330139898326510e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 5.4335870285035925e-09 5.4335870285035925e-01
Overall NLP error.......: 3.6330139898326511e-04 3.6330139898326510e+04
Number of objective function evaluations = 546
Number of objective gradient evaluations = 305
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 547
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 324
Number of Lagrangian Hessian evaluations = 321
Total seconds in IPOPT = 0.344
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.3685479164123535
Solution:
- number of solutions: 0
number of solutions displayed: 0
44 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 629
(scaled) (unscaled)
Objective...............: 2.7317333478079377e-09 2.7317333478079375e-01
Dual infeasibility......: 2.9900452849984329e-09 2.9900452849984327e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6910701327907617e-13 1.6910701327907616e-05
Overall NLP error.......: 2.9900452849984329e-09 2.9900452849984327e-01
Number of objective function evaluations = 3821
Number of objective gradient evaluations = 620
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 3822
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 631
Number of Lagrangian Hessian evaluations = 629
Total seconds in IPOPT = 0.698
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.7223656177520752
Solution:
- number of solutions: 0
number of solutions displayed: 0
45 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 338
(scaled) (unscaled)
Objective...............: 2.9814302667030490e-09 2.9814302667030490e-01
Dual infeasibility......: 1.7769441913995138e-09 1.7769441913995138e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2546273911166799e-13 1.2546273911166799e-05
Overall NLP error.......: 1.7769441913995138e-09 1.7769441913995138e-01
Number of objective function evaluations = 740
Number of objective gradient evaluations = 339
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 741
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 339
Number of Lagrangian Hessian evaluations = 338
Total seconds in IPOPT = 0.376
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3996388912200928
Solution:
- number of solutions: 0
number of solutions displayed: 0
46 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 381
(scaled) (unscaled)
Objective...............: 4.2010958280963333e-09 2.5844950412833245e-01
Dual infeasibility......: 2.0411996497146858e-09 1.2557367384184065e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4777287552899200e-13 9.0909200758202783e-06
Overall NLP error.......: 2.0411996497146858e-09 1.2557367384184065e-01
Number of objective function evaluations = 796
Number of objective gradient evaluations = 382
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 796
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 382
Number of Lagrangian Hessian evaluations = 381
Total seconds in IPOPT = 0.418
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.44257235527038574
Solution:
- number of solutions: 0
number of solutions displayed: 0
47 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 252
(scaled) (unscaled)
Objective...............: 3.6481724184034887e-09 3.6481724184034886e-01
Dual infeasibility......: 4.0477420096896340e-09 4.0477420096896338e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.2473754467413006e-13 3.2473754467413002e-05
Overall NLP error.......: 4.0477420096896340e-09 4.0477420096896338e-01
Number of objective function evaluations = 425
Number of objective gradient evaluations = 253
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 425
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 254
Number of Lagrangian Hessian evaluations = 252
Total seconds in IPOPT = 0.274
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.29854440689086914
Solution:
- number of solutions: 0
number of solutions displayed: 0
48 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 423
(scaled) (unscaled)
Objective...............: 5.0242717661353529e-09 5.0242717661353531e-01
Dual infeasibility......: 8.0056599139232313e-09 8.0056599139232310e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3067719622182898e-13 1.3067719622182897e-05
Overall NLP error.......: 8.0056599139232313e-09 8.0056599139232310e-01
Number of objective function evaluations = 1053
Number of objective gradient evaluations = 424
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1053
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 424
Number of Lagrangian Hessian evaluations = 423
Total seconds in IPOPT = 0.468
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.48868608474731445
Solution:
- number of solutions: 0
number of solutions displayed: 0
49 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 339
(scaled) (unscaled)
Objective...............: 4.0337036034255439e-06 3.1381431446440041e+02
Dual infeasibility......: 4.8670134486971918e-04 3.7864420370273714e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 6.0097395084356071e-09 4.6754607412099963e-01
Overall NLP error.......: 4.8670134486971918e-04 3.7864420370273714e+04
Number of objective function evaluations = 643
Number of objective gradient evaluations = 333
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 643
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 343
Number of Lagrangian Hessian evaluations = 340
Total seconds in IPOPT = 0.367
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.39157986640930176
Solution:
- number of solutions: 0
number of solutions displayed: 0
50 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 347
(scaled) (unscaled)
Objective...............: 3.5647621729207569e-09 3.5647621729207568e-01
Dual infeasibility......: 1.8715498842381903e-09 1.8715498842381903e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2547111933062287e-13 1.2547111933062286e-05
Overall NLP error.......: 1.8715498842381903e-09 1.8715498842381903e-01
Number of objective function evaluations = 713
Number of objective gradient evaluations = 348
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 714
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 348
Number of Lagrangian Hessian evaluations = 347
Total seconds in IPOPT = 0.366
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3899052143096924
Solution:
- number of solutions: 0
number of solutions displayed: 0
51 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 482
(scaled) (unscaled)
Objective...............: 2.9022492346611128e-09 2.9022492346611128e-01
Dual infeasibility......: 2.2096081322540456e-09 1.3596044941111710e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5401715492476289e-13 1.5401715492476288e-05
Overall NLP error.......: 2.2096081322540456e-09 1.3596044941111710e-01
Number of objective function evaluations = 1349
Number of objective gradient evaluations = 478
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1350
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 484
Number of Lagrangian Hessian evaluations = 482
Total seconds in IPOPT = 0.535
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5600457191467285
Solution:
- number of solutions: 0
number of solutions displayed: 0
52 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 466
(scaled) (unscaled)
Objective...............: 5.2583405074427958e-09 5.2583405074427958e-01
Dual infeasibility......: 4.1314045792326297e-09 4.1314045792326298e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.1689909836723670e-13 2.1689909836723670e-05
Overall NLP error.......: 4.1314045792326297e-09 4.1314045792326298e-01
Number of objective function evaluations = 985
Number of objective gradient evaluations = 467
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 985
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 467
Number of Lagrangian Hessian evaluations = 466
Total seconds in IPOPT = 0.492
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5175092220306396
Solution:
- number of solutions: 0
number of solutions displayed: 0
53 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 330
(scaled) (unscaled)
Objective...............: 3.2391526894662656e-09 3.2391526894662653e-01
Dual infeasibility......: 2.6850358771213464e-09 2.6850358771213462e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4013774970251562e-13 1.4013774970251562e-05
Overall NLP error.......: 2.6850358771213464e-09 2.6850358771213462e-01
Number of objective function evaluations = 518
Number of objective gradient evaluations = 323
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 518
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 332
Number of Lagrangian Hessian evaluations = 330
Total seconds in IPOPT = 0.352
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3800673484802246
Solution:
- number of solutions: 0
number of solutions displayed: 0
54 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 300
(scaled) (unscaled)
Objective...............: 3.4264260924076091e-09 3.4264260924076090e-01
Dual infeasibility......: 8.0175448036918592e-07 8.0175448036918596e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4901472982719052e-13 1.4901472982719051e-05
Overall NLP error.......: 8.0175448036918592e-07 8.0175448036918596e+01
Number of objective function evaluations = 584
Number of objective gradient evaluations = 302
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 584
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 302
Number of Lagrangian Hessian evaluations = 301
Total seconds in IPOPT = 0.322
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.3476295471191406
Solution:
- number of solutions: 0
number of solutions displayed: 0
55 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 365
(scaled) (unscaled)
Objective...............: 3.2331846998958241e-09 3.2331846998958241e-01
Dual infeasibility......: 3.4551873862886613e-07 3.4551873862886616e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.9927047977075433e-13 1.9927047977075432e-05
Overall NLP error.......: 3.4551873862886613e-07 3.4551873862886616e+01
Number of objective function evaluations = 904
Number of objective gradient evaluations = 351
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 905
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 370
Number of Lagrangian Hessian evaluations = 366
Total seconds in IPOPT = 0.399
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.423520565032959
Solution:
- number of solutions: 0
number of solutions displayed: 0
56 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 360
(scaled) (unscaled)
Objective...............: 3.0964884620394486e-06 3.0964884620394486e+02
Dual infeasibility......: 3.7572540179136931e-04 3.7572540179136930e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 9.5835491294835181e-09 9.5835491294835173e-01
Overall NLP error.......: 3.7572540179136931e-04 3.7572540179136930e+04
Number of objective function evaluations = 744
Number of objective gradient evaluations = 339
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 744
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 363
Number of Lagrangian Hessian evaluations = 361
Total seconds in IPOPT = 0.380
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.40377020835876465
Solution:
- number of solutions: 0
number of solutions displayed: 0
57 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 397
(scaled) (unscaled)
Objective...............: 1.6950190633610312e-09 1.6950190633610313e-01
Dual infeasibility......: 2.6063762108048001e-09 2.6063762108048000e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2563579840372578e-13 1.2563579840372577e-05
Overall NLP error.......: 2.6063762108048001e-09 2.6063762108048000e-01
Number of objective function evaluations = 630
Number of objective gradient evaluations = 398
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 631
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 398
Number of Lagrangian Hessian evaluations = 397
Total seconds in IPOPT = 0.423
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4469280242919922
Solution:
- number of solutions: 0
number of solutions displayed: 0
58 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 303
(scaled) (unscaled)
Objective...............: 3.9963366752672952e-09 3.9963366752672952e-01
Dual infeasibility......: 5.7404947456593534e-09 5.7404947456593536e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2736133295592734e-13 1.2736133295592733e-05
Overall NLP error.......: 5.7404947456593534e-09 5.7404947456593536e-01
Number of objective function evaluations = 485
Number of objective gradient evaluations = 304
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 486
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 305
Number of Lagrangian Hessian evaluations = 303
Total seconds in IPOPT = 0.332
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.35585451126098633
Solution:
- number of solutions: 0
number of solutions displayed: 0
59 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 338
(scaled) (unscaled)
Objective...............: 2.8663019104528797e-09 2.8663019104528797e-01
Dual infeasibility......: 3.5075746968259942e-09 2.2114928190336364e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4840769760127882e-13 1.4840769760127882e-05
Overall NLP error.......: 3.5075746968259942e-09 2.2114928190336364e-01
Number of objective function evaluations = 511
Number of objective gradient evaluations = 339
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 511
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 339
Number of Lagrangian Hessian evaluations = 338
Total seconds in IPOPT = 0.369
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.39336228370666504
Solution:
- number of solutions: 0
number of solutions displayed: 0
60 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 269
(scaled) (unscaled)
Objective...............: 3.4800165795741188e-09 3.4800165795741189e-01
Dual infeasibility......: 5.4971638302237722e-09 5.4971638302237724e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544303367631448e-13 1.2544303367631448e-05
Overall NLP error.......: 5.4971638302237722e-09 5.4971638302237724e-01
Number of objective function evaluations = 462
Number of objective gradient evaluations = 270
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 462
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 271
Number of Lagrangian Hessian evaluations = 269
Total seconds in IPOPT = 0.297
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3211245536804199
Solution:
- number of solutions: 0
number of solutions displayed: 0
61 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 300
(scaled) (unscaled)
Objective...............: 3.5581730932880663e-09 3.5581730932880662e-01
Dual infeasibility......: 6.9007152988813662e-07 6.9007152988813658e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.7604284524584434e-13 2.7604284524584434e-05
Overall NLP error.......: 6.9007152988813662e-07 6.9007152988813658e+01
Number of objective function evaluations = 608
Number of objective gradient evaluations = 302
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 608
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 302
Number of Lagrangian Hessian evaluations = 301
Total seconds in IPOPT = 0.333
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.3573458194732666
Solution:
- number of solutions: 0
number of solutions displayed: 0
62 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 233
(scaled) (unscaled)
Objective...............: 4.3400569427379529e-06 4.3400569427379526e+02
Dual infeasibility......: 1.1941149761055786e-03 1.1941149761055785e+05
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.3239828097459124e-09 2.3239828097459123e-01
Overall NLP error.......: 1.1941149761055786e-03 1.1941149761055785e+05
Number of objective function evaluations = 322
Number of objective gradient evaluations = 221
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 323
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 236
Number of Lagrangian Hessian evaluations = 234
Total seconds in IPOPT = 0.251
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.27698755264282227
Solution:
- number of solutions: 0
number of solutions displayed: 0
63 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 311
(scaled) (unscaled)
Objective...............: 2.8497960886246459e-09 2.8497960886246460e-01
Dual infeasibility......: 1.6721695415846742e-09 1.6721695415846741e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544316645252029e-13 1.2544316645252028e-05
Overall NLP error.......: 1.6721695415846742e-09 1.6721695415846741e-01
Number of objective function evaluations = 522
Number of objective gradient evaluations = 312
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 522
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 312
Number of Lagrangian Hessian evaluations = 311
Total seconds in IPOPT = 0.342
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.36633920669555664
Solution:
- number of solutions: 0
number of solutions displayed: 0
64 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 485
(scaled) (unscaled)
Objective...............: 4.9361480536029799e-09 4.9361480536029800e-01
Dual infeasibility......: 5.9016702289407439e-09 5.9016702289407441e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3721855813399666e-13 1.3721855813399666e-05
Overall NLP error.......: 5.9016702289407439e-09 5.9016702289407441e-01
Number of objective function evaluations = 1402
Number of objective gradient evaluations = 469
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1403
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 488
Number of Lagrangian Hessian evaluations = 485
Total seconds in IPOPT = 0.521
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5411577224731445
Solution:
- number of solutions: 0
number of solutions displayed: 0
65 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 384
(scaled) (unscaled)
Objective...............: 4.4922626007741724e-09 4.4922626007741723e-01
Dual infeasibility......: 6.8192677493344586e-09 6.8192677493344589e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2741667362752374e-13 1.2741667362752375e-05
Overall NLP error.......: 6.8192677493344586e-09 6.8192677493344589e-01
Number of objective function evaluations = 716
Number of objective gradient evaluations = 385
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 716
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 385
Number of Lagrangian Hessian evaluations = 384
Total seconds in IPOPT = 0.416
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.44066834449768066
Solution:
- number of solutions: 0
number of solutions displayed: 0
66 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 461
(scaled) (unscaled)
Objective...............: 2.7536502162943843e-09 2.7536502162943843e-01
Dual infeasibility......: 4.7941943274133971e-09 4.7941943274133969e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3304391866956622e-13 1.3304391866956622e-05
Overall NLP error.......: 4.7941943274133971e-09 4.7941943274133969e-01
Number of objective function evaluations = 893
Number of objective gradient evaluations = 452
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 893
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 463
Number of Lagrangian Hessian evaluations = 461
Total seconds in IPOPT = 0.508
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5321402549743652
Solution:
- number of solutions: 0
number of solutions displayed: 0
67 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 528
(scaled) (unscaled)
Objective...............: 2.7088345899433226e-06 2.7088345899433227e+02
Dual infeasibility......: 3.5256682781482831e-04 3.5256682781482828e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.7709851846948056e-09 1.7709851846948055e-01
Overall NLP error.......: 3.5256682781482831e-04 3.5256682781482828e+04
Number of objective function evaluations = 3569
Number of objective gradient evaluations = 516
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 3569
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 533
Number of Lagrangian Hessian evaluations = 529
Total seconds in IPOPT = 0.574
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.5996575355529785
Solution:
- number of solutions: 0
number of solutions displayed: 0
68 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 430
(scaled) (unscaled)
Objective...............: 2.7598831231254511e-09 2.7598831231254511e-01
Dual infeasibility......: 5.9726442152333802e-07 5.9726442152333803e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.0807492113422458e-13 2.0807492113422457e-05
Overall NLP error.......: 5.9726442152333802e-07 5.9726442152333803e+01
Number of objective function evaluations = 1901
Number of objective gradient evaluations = 431
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1902
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 431
Number of Lagrangian Hessian evaluations = 430
Total seconds in IPOPT = 0.479
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.5041625499725342
Solution:
- number of solutions: 0
number of solutions displayed: 0
69 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 394
(scaled) (unscaled)
Objective...............: 3.8332163351880736e-09 3.8332163351880733e-01
Dual infeasibility......: 9.6248021877425878e-09 9.6248021877425882e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5133070662637011e-13 1.5133070662637011e-05
Overall NLP error.......: 9.6248021877425878e-09 9.6248021877425882e-01
Number of objective function evaluations = 692
Number of objective gradient evaluations = 395
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 692
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 395
Number of Lagrangian Hessian evaluations = 394
Total seconds in IPOPT = 0.429
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4540586471557617
Solution:
- number of solutions: 0
number of solutions displayed: 0
70 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 357
(scaled) (unscaled)
Objective...............: 3.2274319391760134e-09 3.2274319391760131e-01
Dual infeasibility......: 7.3888466258108987e-09 7.3888466258108987e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2560522119024490e-13 1.2560522119024490e-05
Overall NLP error.......: 7.3888466258108987e-09 7.3888466258108987e-01
Number of objective function evaluations = 734
Number of objective gradient evaluations = 353
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 734
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 359
Number of Lagrangian Hessian evaluations = 357
Total seconds in IPOPT = 0.386
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.41029834747314453
Solution:
- number of solutions: 0
number of solutions displayed: 0
71 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 451
(scaled) (unscaled)
Objective...............: 2.7276052151484919e-09 2.7276052151484920e-01
Dual infeasibility......: 1.8220489689175617e-09 1.8220489689175617e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2559011636801384e-13 1.2559011636801384e-05
Overall NLP error.......: 1.8220489689175617e-09 1.8220489689175617e-01
Number of objective function evaluations = 769
Number of objective gradient evaluations = 452
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 769
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 452
Number of Lagrangian Hessian evaluations = 451
Total seconds in IPOPT = 0.492
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.516157865524292
Solution:
- number of solutions: 0
number of solutions displayed: 0
72 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 299
(scaled) (unscaled)
Objective...............: 2.6776975428786317e-09 2.6776975428786315e-01
Dual infeasibility......: 1.0109170495171341e-09 7.1781786961030103e-02
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544334281346515e-13 1.2544334281346514e-05
Overall NLP error.......: 1.0109170495171341e-09 7.1781786961030103e-02
Number of objective function evaluations = 513
Number of objective gradient evaluations = 300
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 514
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 301
Number of Lagrangian Hessian evaluations = 299
Total seconds in IPOPT = 0.328
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3552820682525635
Solution:
- number of solutions: 0
number of solutions displayed: 0
73 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 238
(scaled) (unscaled)
Objective...............: 4.4713017654980192e-06 4.4713017654980189e+02
Dual infeasibility......: 4.9785089857070372e-04 4.9785089857070372e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6483055571521965e-08 1.6483055571521965e+00
Overall NLP error.......: 4.9785089857070372e-04 4.9785089857070372e+04
Number of objective function evaluations = 342
Number of objective gradient evaluations = 222
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 342
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 241
Number of Lagrangian Hessian evaluations = 239
Total seconds in IPOPT = 0.257
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.28243041038513184
Solution:
- number of solutions: 0
number of solutions displayed: 0
74 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 508
(scaled) (unscaled)
Objective...............: 3.0328260619192422e-09 3.0328260619192421e-01
Dual infeasibility......: 2.4734339208439520e-09 2.4734339208439521e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2633230215011090e-13 1.2633230215011089e-05
Overall NLP error.......: 2.4734339208439520e-09 2.4734339208439521e-01
Number of objective function evaluations = 1672
Number of objective gradient evaluations = 509
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1673
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 511
Number of Lagrangian Hessian evaluations = 508
Total seconds in IPOPT = 0.545
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.5745787620544434
Solution:
- number of solutions: 0
number of solutions displayed: 0
75 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 330
(scaled) (unscaled)
Objective...............: 7.4445718171043553e-09 7.4445718171043551e-01
Dual infeasibility......: 3.4567062297352829e-09 3.4567062297352830e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 9.4050520702956447e-13 9.4050520702956443e-05
Overall NLP error.......: 3.4567062297352829e-09 3.4567062297352830e-01
Number of objective function evaluations = 494
Number of objective gradient evaluations = 331
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 494
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 331
Number of Lagrangian Hessian evaluations = 330
Total seconds in IPOPT = 0.356
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3817586898803711
Solution:
- number of solutions: 0
number of solutions displayed: 0
76 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 361
(scaled) (unscaled)
Objective...............: 6.1432097173178977e-06 6.1432097173178977e+02
Dual infeasibility......: 5.6441578390796553e-04 5.6441578390796552e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.8686525457507863e-09 3.8686525457507864e-01
Overall NLP error.......: 5.6441578390796553e-04 5.6441578390796552e+04
Number of objective function evaluations = 736
Number of objective gradient evaluations = 351
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 736
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 364
Number of Lagrangian Hessian evaluations = 362
Total seconds in IPOPT = 0.384
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.40990328788757324
Solution:
- number of solutions: 0
number of solutions displayed: 0
77 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 292
(scaled) (unscaled)
Objective...............: 3.1694870345774291e-09 2.9889037891302550e-01
Dual infeasibility......: 9.7061970842289407e-09 9.1531812329892615e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4638911238036947e-13 1.3804851318454322e-05
Overall NLP error.......: 9.7061970842289407e-09 9.1531812329892615e-01
Number of objective function evaluations = 540
Number of objective gradient evaluations = 293
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 540
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 293
Number of Lagrangian Hessian evaluations = 292
Total seconds in IPOPT = 0.320
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.34661054611206055
Solution:
- number of solutions: 0
number of solutions displayed: 0
78 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 247
(scaled) (unscaled)
Objective...............: 3.8019057370361996e-06 3.6219803671962984e+02
Dual infeasibility......: 4.1249308231425523e-04 3.9297182757382332e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 4.0686338242051822e-09 3.8760855349532969e-01
Overall NLP error.......: 4.1249308231425523e-04 3.9297182757382332e+04
Number of objective function evaluations = 412
Number of objective gradient evaluations = 242
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 412
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 250
Number of Lagrangian Hessian evaluations = 248
Total seconds in IPOPT = 0.269
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.29744815826416016
Solution:
- number of solutions: 0
number of solutions displayed: 0
79 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 374
(scaled) (unscaled)
Objective...............: 3.5491572112076282e-09 3.5491572112076281e-01
Dual infeasibility......: 3.4641657293594581e-09 3.4641657293594580e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.3962460731008827e-13 1.3962460731008827e-05
Overall NLP error.......: 3.4641657293594581e-09 3.4641657293594580e-01
Number of objective function evaluations = 946
Number of objective gradient evaluations = 375
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 947
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 376
Number of Lagrangian Hessian evaluations = 374
Total seconds in IPOPT = 0.414
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4398820400238037
Solution:
- number of solutions: 0
number of solutions displayed: 0
80 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 310
(scaled) (unscaled)
Objective...............: 4.2339236404057469e-09 4.2339236404057468e-01
Dual infeasibility......: 1.1526693774895933e-09 1.1526693774895934e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4433722174250404e-13 1.4433722174250404e-05
Overall NLP error.......: 1.1526693774895933e-09 1.1526693774895934e-01
Number of objective function evaluations = 461
Number of objective gradient evaluations = 311
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 462
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 311
Number of Lagrangian Hessian evaluations = 310
Total seconds in IPOPT = 0.325
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.34898877143859863
Solution:
- number of solutions: 0
number of solutions displayed: 0
81 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 340
(scaled) (unscaled)
Objective...............: 2.0899998690373695e-09 2.0899998690373695e-01
Dual infeasibility......: 9.8170777546381625e-09 9.8170777546381627e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544455621972793e-13 1.2544455621972793e-05
Overall NLP error.......: 9.8170777546381625e-09 9.8170777546381627e-01
Number of objective function evaluations = 629
Number of objective gradient evaluations = 341
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 630
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 341
Number of Lagrangian Hessian evaluations = 340
Total seconds in IPOPT = 0.368
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3869946002960205
Solution:
- number of solutions: 0
number of solutions displayed: 0
82 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 435
(scaled) (unscaled)
Objective...............: 6.9810586680405877e-06 6.9810586680405879e+02
Dual infeasibility......: 6.2029061456045571e-04 6.2029061456045572e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 4.5517018580853721e-09 4.5517018580853719e-01
Overall NLP error.......: 6.2029061456045571e-04 6.2029061456045572e+04
Number of objective function evaluations = 1697
Number of objective gradient evaluations = 425
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1698
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 438
Number of Lagrangian Hessian evaluations = 436
Total seconds in IPOPT = 0.468
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.4929769039154053
Solution:
- number of solutions: 0
number of solutions displayed: 0
83 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 377
(scaled) (unscaled)
Objective...............: 3.3434168855276214e-09 3.3434168855276214e-01
Dual infeasibility......: 4.6473409503418528e-09 1.9622465402400688e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.1439447098276044e-13 2.1439447098276044e-05
Overall NLP error.......: 4.6473409503418528e-09 1.9622465402400688e-01
Number of objective function evaluations = 715
Number of objective gradient evaluations = 378
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 716
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 378
Number of Lagrangian Hessian evaluations = 377
Total seconds in IPOPT = 0.419
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.44387149810791016
Solution:
- number of solutions: 0
number of solutions displayed: 0
84 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 297
(scaled) (unscaled)
Objective...............: 4.1154159200784901e-06 4.1154159200784898e+02
Dual infeasibility......: 4.2175515875507750e-04 4.2175515875507750e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6897153353608675e-08 1.6897153353608676e+00
Overall NLP error.......: 4.2175515875507750e-04 4.2175515875507750e+04
Number of objective function evaluations = 478
Number of objective gradient evaluations = 286
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 478
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 300
Number of Lagrangian Hessian evaluations = 298
Total seconds in IPOPT = 0.325
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.34889936447143555
Solution:
- number of solutions: 0
number of solutions displayed: 0
85 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 324
(scaled) (unscaled)
Objective...............: 4.9231273728528121e-09 4.9231273728528119e-01
Dual infeasibility......: 4.7601107914442284e-09 4.6432796964343281e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.9518737399101712e-13 3.9518737399101711e-05
Overall NLP error.......: 4.7601107914442284e-09 4.6432796964343281e-01
Number of objective function evaluations = 592
Number of objective gradient evaluations = 325
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 593
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 325
Number of Lagrangian Hessian evaluations = 324
Total seconds in IPOPT = 0.348
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3722515106201172
Solution:
- number of solutions: 0
number of solutions displayed: 0
86 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 383
(scaled) (unscaled)
Objective...............: 3.5940960519807251e-09 3.5940960519807252e-01
Dual infeasibility......: 3.2846303635423682e-07 3.2846303635423681e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.5059208146669621e-13 1.5059208146669621e-05
Overall NLP error.......: 3.2846303635423682e-07 3.2846303635423681e+01
Number of objective function evaluations = 758
Number of objective gradient evaluations = 385
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 759
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 385
Number of Lagrangian Hessian evaluations = 384
Total seconds in IPOPT = 0.427
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.45122289657592773
Solution:
- number of solutions: 0
number of solutions displayed: 0
87 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 329
(scaled) (unscaled)
Objective...............: 2.0516753855033660e-09 2.0516753855033659e-01
Dual infeasibility......: 3.1698522090277467e-09 3.1698522090277464e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2579315041603952e-13 1.2579315041603952e-05
Overall NLP error.......: 3.1698522090277467e-09 3.1698522090277464e-01
Number of objective function evaluations = 751
Number of objective gradient evaluations = 330
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 751
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 330
Number of Lagrangian Hessian evaluations = 329
Total seconds in IPOPT = 0.364
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.3885188102722168
Solution:
- number of solutions: 0
number of solutions displayed: 0
88 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 314
(scaled) (unscaled)
Objective...............: 2.0840456816227127e-09 2.0840456816227126e-01
Dual infeasibility......: 3.4824537213797519e-09 3.4824537213797518e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544303203870740e-13 1.2544303203870740e-05
Overall NLP error.......: 3.4824537213797519e-09 3.4824537213797518e-01
Number of objective function evaluations = 567
Number of objective gradient evaluations = 315
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 568
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 315
Number of Lagrangian Hessian evaluations = 314
Total seconds in IPOPT = 0.340
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.35916805267333984
Solution:
- number of solutions: 0
number of solutions displayed: 0
89 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 395
(scaled) (unscaled)
Objective...............: 2.8053390358209256e-09 2.8053390358209257e-01
Dual infeasibility......: 3.1067371489493370e-09 1.3243392940288368e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.6438976978859477e-13 1.6438976978859477e-05
Overall NLP error.......: 3.1067371489493370e-09 1.3243392940288368e-01
Number of objective function evaluations = 601
Number of objective gradient evaluations = 396
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 601
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 396
Number of Lagrangian Hessian evaluations = 395
Total seconds in IPOPT = 0.426
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4500393867492676
Solution:
- number of solutions: 0
number of solutions displayed: 0
90 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 300
(scaled) (unscaled)
Objective...............: 8.0484806354133040e-09 8.0484806354133043e-01
Dual infeasibility......: 9.0826407227050480e-09 9.0826407227050476e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 9.4283108862623443e-13 9.4283108862623443e-05
Overall NLP error.......: 9.0826407227050480e-09 9.0826407227050476e-01
Number of objective function evaluations = 565
Number of objective gradient evaluations = 292
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 565
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 302
Number of Lagrangian Hessian evaluations = 300
Total seconds in IPOPT = 0.328
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.356703519821167
Solution:
- number of solutions: 0
number of solutions displayed: 0
91 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 470
(scaled) (unscaled)
Objective...............: 3.3107048782911991e-09 3.3107048782911991e-01
Dual infeasibility......: 6.4669988727605947e-07 6.4669988727605954e+01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.8098671806975983e-13 1.8098671806975984e-05
Overall NLP error.......: 6.4669988727605947e-07 6.4669988727605954e+01
Number of objective function evaluations = 1065
Number of objective gradient evaluations = 472
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1065
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 472
Number of Lagrangian Hessian evaluations = 471
Total seconds in IPOPT = 0.510
EXIT: Solved To Acceptable Level.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Solved To Acceptable Level.
Termination condition: optimal
Id: 1
Error rc: 0
Time: 0.5344500541687012
Solution:
- number of solutions: 0
number of solutions displayed: 0
92 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 313
(scaled) (unscaled)
Objective...............: 5.4509024931684988e-06 5.4509024931684985e+02
Dual infeasibility......: 5.3288829076146192e-04 5.3288829076146190e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 3.3039529623111930e-09 3.3039529623111930e-01
Overall NLP error.......: 5.3288829076146192e-04 5.3288829076146190e+04
Number of objective function evaluations = 790
Number of objective gradient evaluations = 308
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 790
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 316
Number of Lagrangian Hessian evaluations = 314
Total seconds in IPOPT = 0.335
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.3589444160461426
Solution:
- number of solutions: 0
number of solutions displayed: 0
93 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 351
(scaled) (unscaled)
Objective...............: 3.5711720686050729e-09 3.5711720686050730e-01
Dual infeasibility......: 3.2690922833975850e-09 3.2690922833975850e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2546537559728400e-13 1.2546537559728400e-05
Overall NLP error.......: 3.2690922833975850e-09 3.2690922833975850e-01
Number of objective function evaluations = 670
Number of objective gradient evaluations = 345
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 670
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 353
Number of Lagrangian Hessian evaluations = 351
Total seconds in IPOPT = 0.381
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.40584707260131836
Solution:
- number of solutions: 0
number of solutions displayed: 0
94 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 338
(scaled) (unscaled)
Objective...............: 3.1474726582253762e-09 3.1474726582253759e-01
Dual infeasibility......: 1.9997475019956302e-09 1.9997475019956301e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.4396733191027071e-13 1.4396733191027071e-05
Overall NLP error.......: 1.9997475019956302e-09 1.9997475019956301e-01
Number of objective function evaluations = 817
Number of objective gradient evaluations = 339
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 817
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 339
Number of Lagrangian Hessian evaluations = 338
Total seconds in IPOPT = 0.367
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.39171695709228516
Solution:
- number of solutions: 0
number of solutions displayed: 0
95 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 302
(scaled) (unscaled)
Objective...............: 3.2832065208704154e-09 3.2832065208704153e-01
Dual infeasibility......: 7.2397779350788686e-10 7.2397779350788685e-02
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2628663709803933e-13 1.2628663709803934e-05
Overall NLP error.......: 7.2397779350788686e-10 7.2397779350788685e-02
Number of objective function evaluations = 530
Number of objective gradient evaluations = 303
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 531
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 303
Number of Lagrangian Hessian evaluations = 302
Total seconds in IPOPT = 0.331
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.35486936569213867
Solution:
- number of solutions: 0
number of solutions displayed: 0
96 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 292
(scaled) (unscaled)
Objective...............: 3.1842539436206512e-09 3.1842539436206513e-01
Dual infeasibility......: 5.1480781543306637e-09 5.1480781543306642e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2544314439843232e-13 1.2544314439843231e-05
Overall NLP error.......: 5.1480781543306637e-09 5.1480781543306642e-01
Number of objective function evaluations = 559
Number of objective gradient evaluations = 284
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 560
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 294
Number of Lagrangian Hessian evaluations = 292
Total seconds in IPOPT = 0.325
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.34821534156799316
Solution:
- number of solutions: 0
number of solutions displayed: 0
97 ok optimal
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 381
(scaled) (unscaled)
Objective...............: 3.8817930645203892e-06 3.8817930645203893e+02
Dual infeasibility......: 4.6011546632181117e-04 4.6011546632181118e+04
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 2.1531274316507906e-09 2.1531274316507906e-01
Overall NLP error.......: 4.6011546632181117e-04 4.6011546632181118e+04
Number of objective function evaluations = 1052
Number of objective gradient evaluations = 367
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 1053
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 385
Number of Lagrangian Hessian evaluations = 382
Total seconds in IPOPT = 0.442
EXIT: Converged to a point of local infeasibility. Problem may be infeasible.
WARNING: Loading a SolverResults object with a warning status into
model.name="iGFA";
- termination condition: infeasible
- message from solver: Ipopt 3.14.16\x3a Converged to a locally infeasible
point. Problem may be infeasible.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: warning
Message: Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible.
Termination condition: infeasible
Id: 200
Error rc: 0
Time: 0.4676368236541748
Solution:
- number of solutions: 0
number of solutions displayed: 0
98 warning infeasible
------------------------------------------------------------------------------
Ipopt 3.14.16: max_iter=100000
linear_solver=mumps
warm_start_init_point=yes
print_level=4
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit https://github.com/coin-or/Ipopt
******************************************************************************
Total number of variables............................: 70
variables with only lower bounds: 12
variables with lower and upper bounds: 58
variables with only upper bounds: 0
Total number of equality constraints.................: 0
Total number of inequality constraints...............: 53
inequality constraints with only lower bounds: 52
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 1
Number of Iterations....: 364
(scaled) (unscaled)
Objective...............: 3.1296560275211738e-09 3.1296560275211738e-01
Dual infeasibility......: 7.1871330280299137e-09 7.1871330280299139e-01
Constraint violation....: 0.0000000000000000e+00 0.0000000000000000e+00
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.2567224828658512e-13 1.2567224828658512e-05
Overall NLP error.......: 7.1871330280299137e-09 7.1871330280299139e-01
Number of objective function evaluations = 782
Number of objective gradient evaluations = 365
Number of equality constraint evaluations = 0
Number of inequality constraint evaluations = 782
Number of equality constraint Jacobian evaluations = 0
Number of inequality constraint Jacobian evaluations = 365
Number of Lagrangian Hessian evaluations = 364
Total seconds in IPOPT = 0.399
EXIT: Optimal Solution Found.
Problem:
- Lower bound: -inf
Upper bound: inf
Number of objectives: 1
Number of constraints: 53
Number of variables: 84
Sense: unknown
Solver:
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4233970642089844
Solution:
- number of solutions: 0
number of solutions displayed: 0
99 ok optimal
Save results¶
# pickle_out = open(os.path.join(mainDir, 'results', 'Lee et al', pH_scale+'_fitlux_fitbeta.pickle'), 'wb')
# pickle.dump(results_dict, pickle_out)
# pickle_out.close()
Visualization¶
res_data = res_plotter(results_dict, multi_result=True, met_meta=met_meta, rxn_meta=rxn_meta)
display(res_data.summarize_runs())
Show code cell output
Runs | fit_betas | fit_flux | fit_spec_prod | obj | min_params | message |
---|---|---|---|---|---|---|
58 | 0.038964 | 0.106549 | 0.023989 | 0.169502 | 68.599830 | Ipopt 3.14.16\x3a Optimal Solution Found |
16 | 0.042025 | 0.117618 | 0.024711 | 0.184354 | 68.713055 | Ipopt 3.14.16\x3a Optimal Solution Found |
34 | 0.040866 | 0.125645 | 0.024549 | 0.191060 | 68.529072 | Ipopt 3.14.16\x3a Optimal Solution Found |
25 | 0.046068 | 0.122862 | 0.025267 | 0.194197 | 84.724603 | Ipopt 3.14.16\x3a Optimal Solution Found |
88 | 0.047540 | 0.132617 | 0.025011 | 0.205168 | 69.192132 | Ipopt 3.14.16\x3a Optimal Solution Found |
24 | 0.044848 | 0.136617 | 0.025258 | 0.206723 | 68.765661 | Ipopt 3.14.16\x3a Optimal Solution Found |
89 | 0.047693 | 0.136761 | 0.023950 | 0.208405 | 68.775684 | Ipopt 3.14.16\x3a Optimal Solution Found |
82 | 0.049582 | 0.134569 | 0.024849 | 0.209000 | 68.562238 | Ipopt 3.14.16\x3a Optimal Solution Found |
5 | 0.047937 | 0.144373 | 0.026489 | 0.218799 | 68.376858 | Ipopt 3.14.16\x3a Optimal Solution Found |
47 | 0.052234 | 0.181607 | 0.024608 | 0.258450 | 68.362714 | Ipopt 3.14.16\x3a Optimal Solution Found |
15 | 0.053066 | 0.193498 | 0.017373 | 0.263937 | 68.556723 | Ipopt 3.14.16\x3a Optimal Solution Found |
73 | 0.053224 | 0.182050 | 0.032496 | 0.267770 | 67.994763 | Ipopt 3.14.16\x3a Optimal Solution Found |
72 | 0.056774 | 0.190616 | 0.025370 | 0.272761 | 68.710712 | Ipopt 3.14.16\x3a Optimal Solution Found |
37 | 0.056140 | 0.188700 | 0.028095 | 0.272936 | 68.035200 | Ipopt 3.14.16\x3a Optimal Solution Found |
45 | 0.063446 | 0.180919 | 0.028809 | 0.273173 | 68.379803 | Ipopt 3.14.16\x3a Optimal Solution Found |
3 | 0.058104 | 0.190373 | 0.025841 | 0.274318 | 68.574487 | Ipopt 3.14.16\x3a Optimal Solution Found |
67 | 0.053562 | 0.196129 | 0.025674 | 0.275365 | 68.310518 | Ipopt 3.14.16\x3a Optimal Solution Found |
69 | 0.058784 | 0.191430 | 0.025774 | 0.275988 | 68.544281 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
6 | 0.057840 | 0.195553 | 0.025694 | 0.279086 | 68.528285 | Ipopt 3.14.16\x3a Optimal Solution Found |
90 | 0.049104 | 0.202985 | 0.028445 | 0.280534 | 67.840712 | Ipopt 3.14.16\x3a Optimal Solution Found |
64 | 0.055426 | 0.205368 | 0.024186 | 0.284980 | 68.698178 | Ipopt 3.14.16\x3a Optimal Solution Found |
60 | 0.056152 | 0.206769 | 0.023709 | 0.286630 | 68.658572 | Ipopt 3.14.16\x3a Optimal Solution Found |
43 | 0.056351 | 0.211394 | 0.021583 | 0.289328 | 68.520120 | Ipopt 3.14.16\x3a Optimal Solution Found |
52 | 0.058451 | 0.206273 | 0.025501 | 0.290225 | 68.450284 | Ipopt 3.14.16\x3a Optimal Solution Found |
46 | 0.056757 | 0.209465 | 0.031921 | 0.298143 | 71.229079 | Ipopt 3.14.16\x3a Optimal Solution Found |
78 | 0.045168 | 0.234188 | 0.019534 | 0.298890 | 68.183671 | Ipopt 3.14.16\x3a Optimal Solution Found |
33 | 0.049005 | 0.232485 | 0.020466 | 0.301956 | 67.816796 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
75 | 0.059338 | 0.214782 | 0.029162 | 0.303283 | 67.656625 | Ipopt 3.14.16\x3a Optimal Solution Found |
30 | 0.056285 | 0.228212 | 0.020477 | 0.304974 | 68.623696 | Ipopt 3.14.16\x3a Optimal Solution Found |
7 | 0.054799 | 0.230781 | 0.020172 | 0.305752 | 68.462136 | Ipopt 3.14.16\x3a Optimal Solution Found |
12 | 0.049422 | 0.239511 | 0.020974 | 0.309907 | 66.268271 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
99 | 0.050310 | 0.240533 | 0.022123 | 0.312966 | 68.948193 | Ipopt 3.14.16\x3a Optimal Solution Found |
95 | 0.049129 | 0.244308 | 0.021311 | 0.314747 | 67.772573 | Ipopt 3.14.16\x3a Optimal Solution Found |
97 | 0.059988 | 0.233952 | 0.024485 | 0.318425 | 68.183401 | Ipopt 3.14.16\x3a Optimal Solution Found |
26 | 0.061521 | 0.229832 | 0.027638 | 0.318992 | 66.315274 | Ipopt 3.14.16\x3a Optimal Solution Found |
17 | 0.049923 | 0.253764 | 0.016590 | 0.320277 | 68.463317 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
71 | 0.048339 | 0.255144 | 0.019260 | 0.322743 | 68.356094 | Ipopt 3.14.16\x3a Optimal Solution Found |
56 | 0.053764 | 0.248249 | 0.021306 | 0.323318 | 68.332258 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
54 | 0.055414 | 0.246075 | 0.022427 | 0.323915 | 68.471910 | Ipopt 3.14.16\x3a Optimal Solution Found |
96 | 0.056215 | 0.242505 | 0.029601 | 0.328321 | 68.260187 | Ipopt 3.14.16\x3a Optimal Solution Found |
92 | 0.056777 | 0.252286 | 0.022007 | 0.331070 | 68.493473 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
35 | 0.059935 | 0.244818 | 0.027673 | 0.332426 | 68.531205 | Ipopt 3.14.16\x3a Optimal Solution Found |
84 | 0.054529 | 0.256154 | 0.023658 | 0.334342 | 68.502741 | Ipopt 3.14.16\x3a Optimal Solution Found |
21 | 0.045742 | 0.275328 | 0.018537 | 0.339607 | 68.445772 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
55 | 0.053368 | 0.271209 | 0.018066 | 0.342643 | 68.496166 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
32 | 0.053034 | 0.266511 | 0.024008 | 0.343553 | 68.273320 | Ipopt 3.14.16\x3a Optimal Solution Found |
39 | 0.047781 | 0.277955 | 0.020285 | 0.346022 | 68.473385 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
61 | 0.043975 | 0.278165 | 0.025861 | 0.348002 | 67.455558 | Ipopt 3.14.16\x3a Optimal Solution Found |
28 | 0.056308 | 0.265572 | 0.029332 | 0.351213 | 68.523097 | Ipopt 3.14.16\x3a Optimal Solution Found |
19 | 0.046408 | 0.285895 | 0.019085 | 0.351388 | 68.512391 | Ipopt 3.14.16\x3a Optimal Solution Found |
38 | 0.043819 | 0.277794 | 0.030401 | 0.352015 | 66.409670 | Ipopt 3.14.16\x3a Optimal Solution Found |
80 | 0.051543 | 0.283279 | 0.020094 | 0.354916 | 68.552513 | Ipopt 3.14.16\x3a Optimal Solution Found |
22 | 0.042825 | 0.289642 | 0.022635 | 0.355102 | 68.397202 | Ipopt 3.14.16\x3a Optimal Solution Found |
62 | 0.052687 | 0.286332 | 0.016798 | 0.355817 | 68.547235 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
51 | 0.056969 | 0.273856 | 0.025651 | 0.356476 | 68.464066 | Ipopt 3.14.16\x3a Optimal Solution Found |
94 | 0.052617 | 0.278732 | 0.025768 | 0.357117 | 68.205924 | Ipopt 3.14.16\x3a Optimal Solution Found |
87 | 0.048517 | 0.292186 | 0.018707 | 0.359410 | 68.434467 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
10 | 0.049404 | 0.294561 | 0.017423 | 0.361388 | 68.449345 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
14 | 0.041117 | 0.301343 | 0.019974 | 0.362434 | 68.525798 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
8 | 0.047769 | 0.297923 | 0.018566 | 0.364258 | 68.557410 | Ipopt 3.14.16\x3a Optimal Solution Found |
48 | 0.048114 | 0.297994 | 0.018709 | 0.364817 | 68.152472 | Ipopt 3.14.16\x3a Optimal Solution Found |
70 | 0.048022 | 0.315296 | 0.020005 | 0.383322 | 68.456714 | Ipopt 3.14.16\x3a Optimal Solution Found |
23 | 0.062868 | 0.311929 | 0.018944 | 0.393740 | 68.405972 | Ipopt 3.14.16\x3a Optimal Solution Found |
20 | 0.075873 | 0.300241 | 0.019939 | 0.396053 | 68.417017 | Ipopt 3.14.16\x3a Optimal Solution Found |
42 | 0.077039 | 0.298184 | 0.021054 | 0.396277 | 68.280266 | Ipopt 3.14.16\x3a Optimal Solution Found |
59 | 0.048641 | 0.285145 | 0.065848 | 0.399634 | 65.373918 | Ipopt 3.14.16\x3a Optimal Solution Found |
81 | 0.079397 | 0.323954 | 0.020041 | 0.423392 | 68.411735 | Ipopt 3.14.16\x3a Optimal Solution Found |
66 | 0.098157 | 0.326186 | 0.024883 | 0.449226 | 68.387005 | Ipopt 3.14.16\x3a Optimal Solution Found |
86 | 0.090302 | 0.377994 | 0.024016 | 0.492313 | 68.367355 | Ipopt 3.14.16\x3a Optimal Solution Found |
65 | 0.107231 | 0.362882 | 0.023502 | 0.493615 | 68.324253 | Ipopt 3.14.16\x3a Optimal Solution Found |
18 | 0.124412 | 0.347981 | 0.027023 | 0.499416 | 68.442922 | Ipopt 3.14.16\x3a Solved To Acceptable Level. |
49 | 0.118232 | 0.358485 | 0.025710 | 0.502427 | 67.754980 | Ipopt 3.14.16\x3a Optimal Solution Found |
9 | 0.114534 | 0.367212 | 0.028592 | 0.510338 | 68.048715 | Ipopt 3.14.16\x3a Optimal Solution Found |
53 | 0.094160 | 0.408606 | 0.023068 | 0.525834 | 68.426091 | Ipopt 3.14.16\x3a Optimal Solution Found |
76 | 0.254195 | 0.454396 | 0.035866 | 0.744457 | 68.362288 | Ipopt 3.14.16\x3a Optimal Solution Found |
41 | 0.258446 | 0.458091 | 0.041302 | 0.757840 | 68.138641 | Ipopt 3.14.16\x3a Optimal Solution Found |
91 | 0.217456 | 0.505432 | 0.081960 | 0.804848 | 66.839777 | Ipopt 3.14.16\x3a Optimal Solution Found |
0 | 0.069904 | 127.001679 | 0.066529 | 127.138112 | 40.034724 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
68 | 0.795017 | 269.956568 | 0.131875 | 270.883459 | 66.697377 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
31 | 0.770668 | 277.050831 | 0.953793 | 278.775291 | 66.872357 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
40 | 0.813956 | 280.277295 | 0.121654 | 281.212905 | 67.679053 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
1 | 0.344394 | 305.453593 | 0.063712 | 305.861699 | 68.299858 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
57 | 0.340179 | 309.233336 | 0.075331 | 309.648846 | 68.529457 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
50 | 0.728043 | 312.977841 | 0.108431 | 313.814314 | 67.712836 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
44 | 0.346279 | 314.234411 | 0.072887 | 314.653576 | 68.559452 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
79 | 0.870788 | 361.172792 | 0.154456 | 362.198037 | 66.938769 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
98 | 0.924783 | 387.126736 | 0.127787 | 388.179306 | 68.242717 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
4 | 0.482047 | 394.756520 | 0.099920 | 395.338487 | 68.432761 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
85 | 0.972169 | 409.972165 | 0.597257 | 411.541592 | 65.311769 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
63 | 1.312267 | 431.966654 | 0.726773 | 434.005694 | 85.165110 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
74 | 1.106714 | 442.731974 | 3.291488 | 447.130177 | 70.985508 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
2 | 1.116287 | 500.957250 | 0.199100 | 502.272638 | 65.075450 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
93 | 1.105509 | 543.834011 | 0.150730 | 545.090249 | 67.546800 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
13 | 1.126825 | 558.540397 | 0.198357 | 559.865579 | 65.860093 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
29 | 1.156050 | 568.759235 | 0.189839 | 570.105124 | 67.765885 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
27 | 1.186158 | 587.985494 | 0.183529 | 589.355181 | 66.753797 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
77 | 1.140915 | 613.003252 | 0.176805 | 614.320972 | 67.783945 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
83 | 1.272586 | 696.656276 | 0.177005 | 698.105867 | 68.163689 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
11 | 1.232298 | 709.665890 | 0.167944 | 711.066132 | 68.146790 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
36 | 3.999853 | 1325.132178 | 44.219507 | 1373.351539 | 67.302621 | Ipopt 3.14.16\x3a Converged to a locally infeasible point. Problem may be infeasible. |
res_data.sel_result(58)
print(res_data)
- Status: ok
Message: Ipopt 3.14.16\x3a Optimal Solution Found
Termination condition: optimal
Id: 0
Error rc: 0
Time: 0.4469280242919922
Results class (name=None) with 100 experiments (Active=58):
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99
top_n_results = res_data.choose_top_n('obj', 10, ascending=True)
Secreted Fluxes¶
fig = res_data.plot_secretedflux(time_col= 'Time (WD)', meas_cols=None, ncols=5, figsize=None, res_ids=top_n_results[0:2], smooth_kwargs={'markersize': 0})
{'markersize': 0}
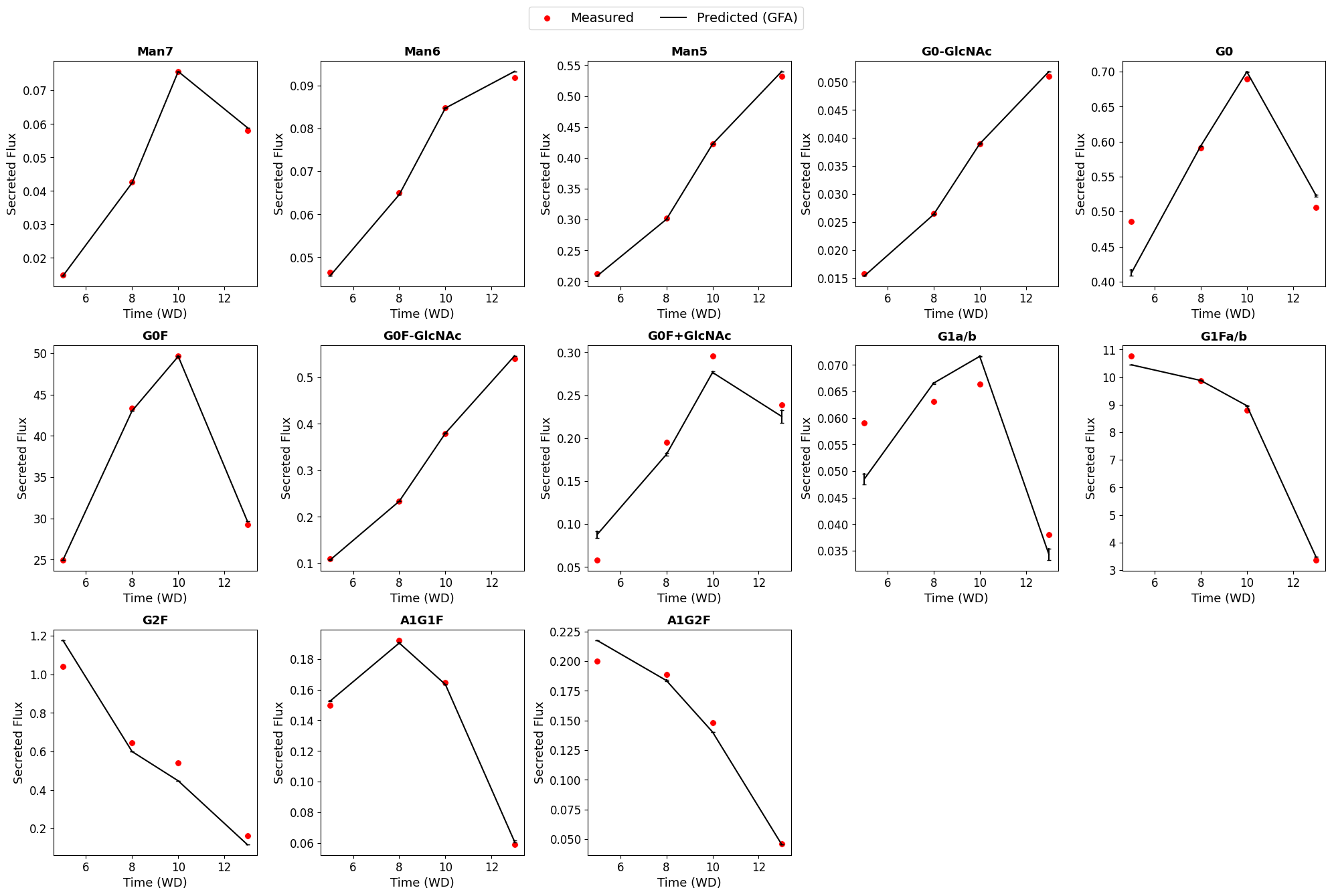
Alpha¶
fig = res_data.plot_alphas(time_col='Time (WD)', meas_cols=None, ncols=5, res_ids=top_n_results, figsize=None)
{}
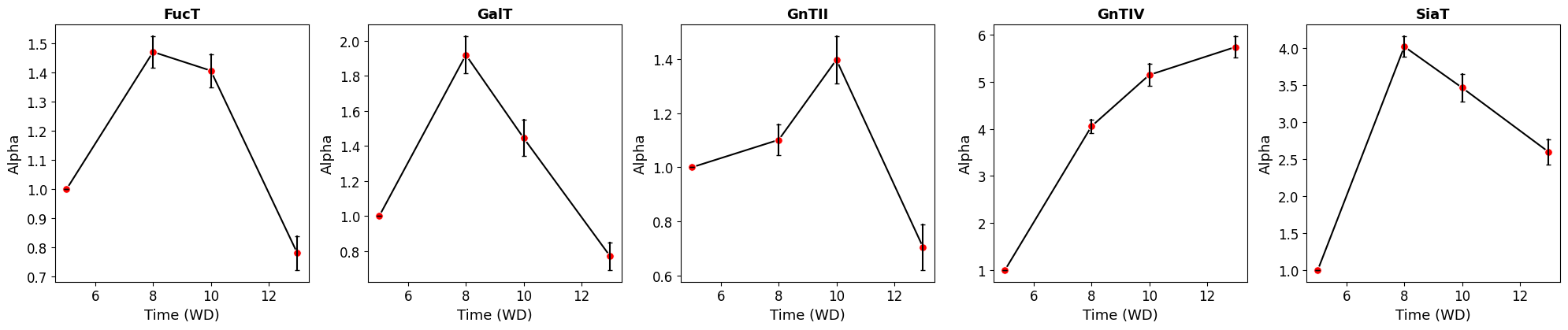
Beta¶
fig = res_data.plot_betas(time_col = 'Time (WD)', meas_cols=None, ncols=5, figsize=None, res_ids=top_n_results)
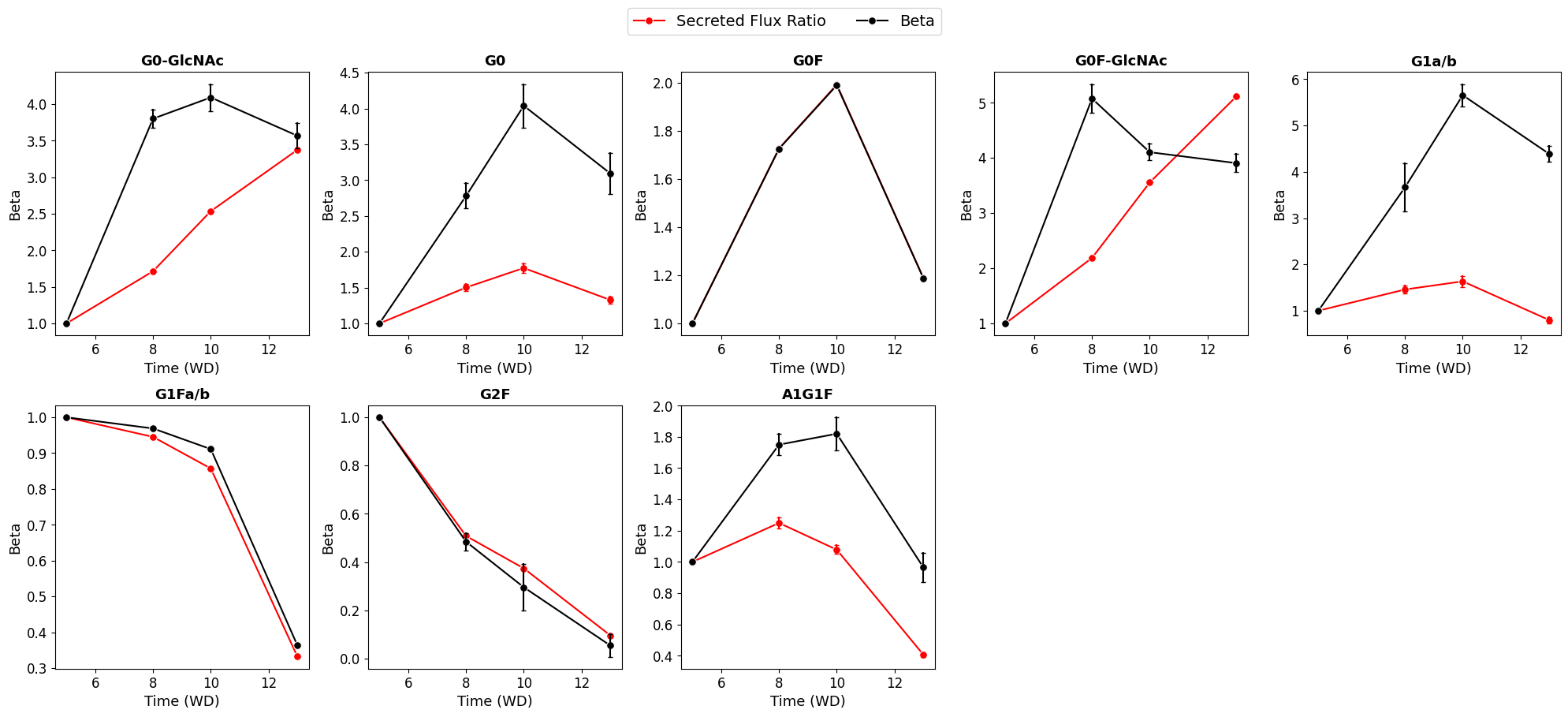
Gamma¶
fig = res_data.plot_gamma(time_col = 'Time (WD)', meas_cols=None, ncols=5, figsize=None, res_ids=top_n_results)
{}
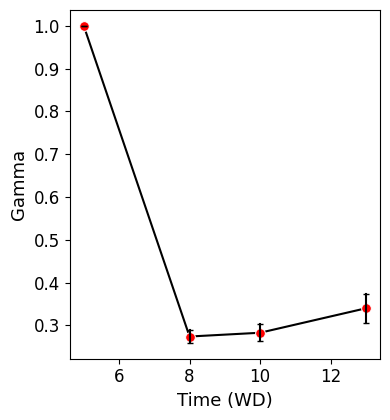
Entry Flux¶
fig = res_data.plot_entry_flux(time_col='Time (WD)', meas_cols=None, ncols=5, figsize=None, res_ids=top_n_results)
sns.lineplot(init_model.spec_prod, x='Time (WD)', y='Spec Prod (pg/cells/day)', c='g', linestyle='-.', ax=fig.axes[0])
tick_mins = np.floor(init_model.spec_prod['Spec Prod (pg/cells/day)'].min()) - 5
tick_maxs = np.ceil(init_model.spec_prod['Spec Prod (pg/cells/day)'].max()) + 5
fig.axes[0].set_yticks(np.arange(tick_mins, tick_maxs, 5))
{}
[<matplotlib.axis.YTick at 0x7f362d6aedd0>,
<matplotlib.axis.YTick at 0x7f362d799f10>,
<matplotlib.axis.YTick at 0x7f362db30510>,
<matplotlib.axis.YTick at 0x7f362d42bf50>,
<matplotlib.axis.YTick at 0x7f362d420550>,
<matplotlib.axis.YTick at 0x7f362d6bca50>,
<matplotlib.axis.YTick at 0x7f362d6dd8d0>,
<matplotlib.axis.YTick at 0x7f362d435a10>]
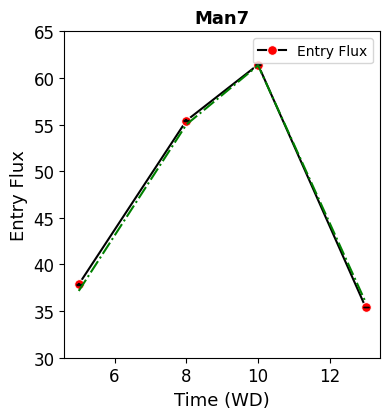
Reference Fluxes¶
fig = res_data.plot_vref(x_col='Reaction ID', meas_cols=None, ncols=5, figsize=(5, 5), res_ids=top_n_results)
plt.setp(fig.axes[0].get_xticklabels(), fontsize=10)
plt.show()
{}
[None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None]
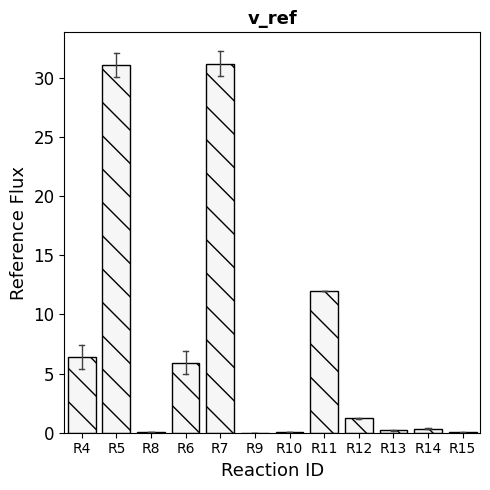
Internal Fluxes¶
fig = res_data.plot_internalflux(time_col='Time (WD)', meas_cols=None, ncols=5, figsize=None, res_ids=top_n_results)
{}
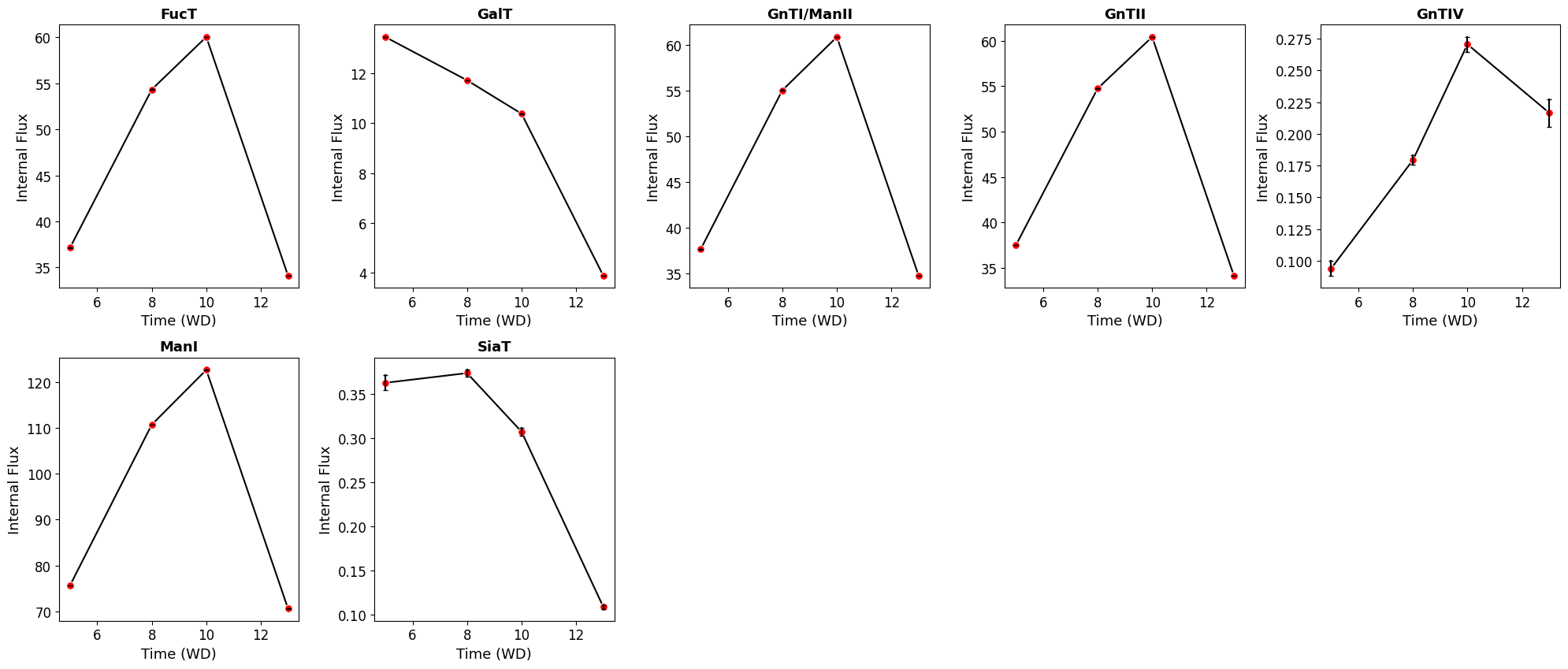